Last Updated or created 2022-11-02
Coline sometimes plays games with her sisters online using my streaming server. (Due to Covid)
Some games are difficult because you have to point to a location on the table. So i came up with a laser pointer solution.
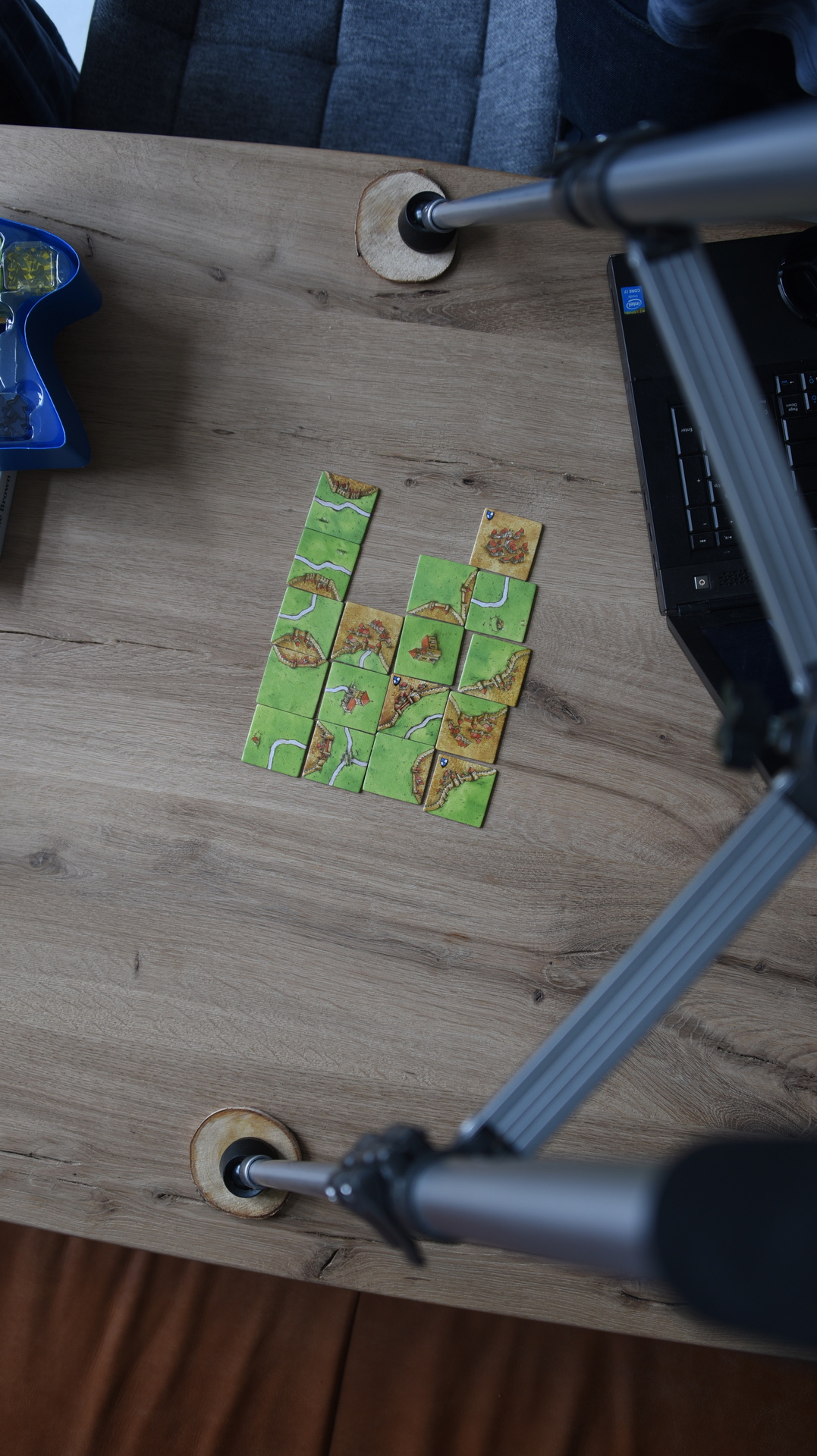
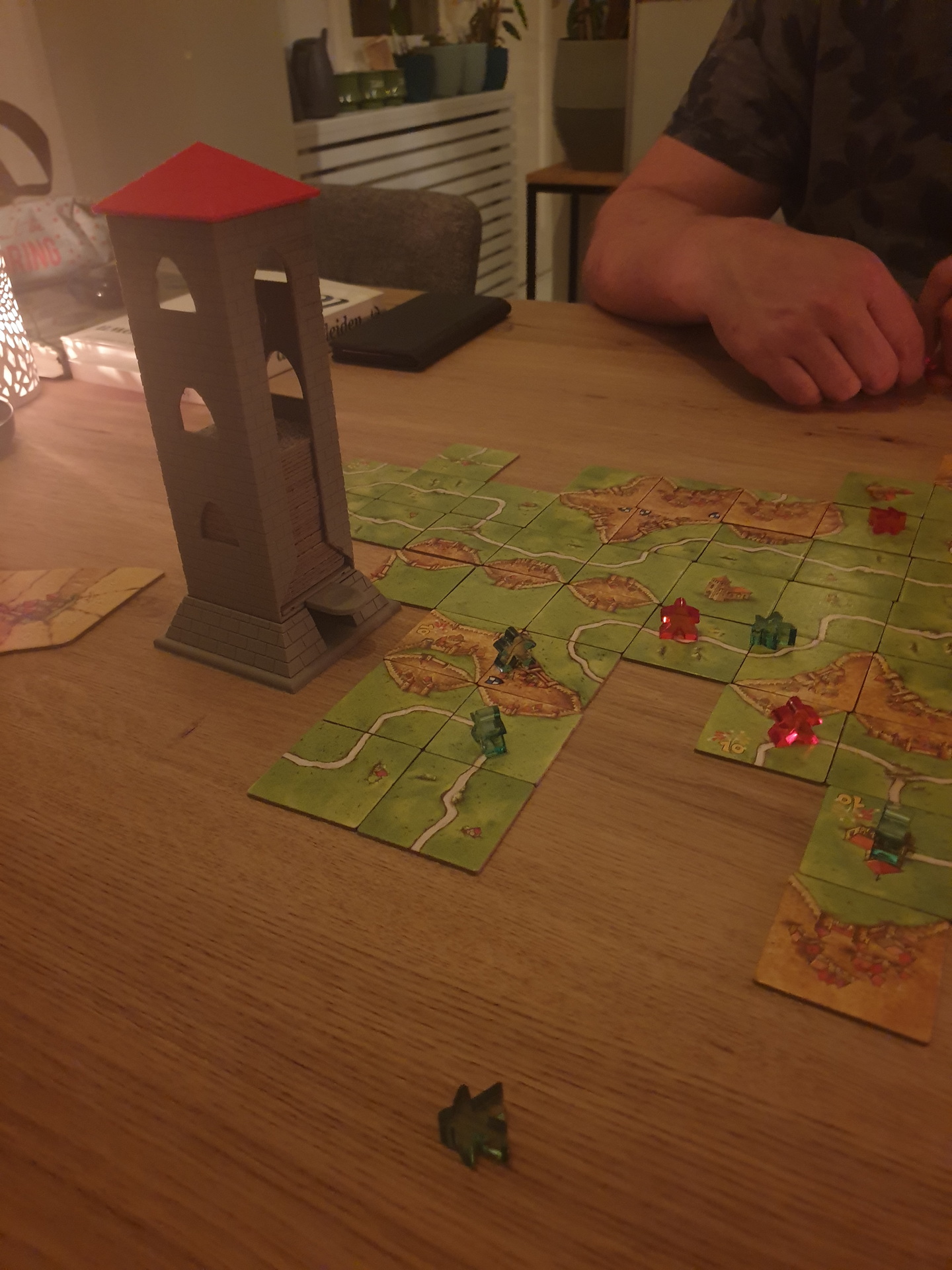
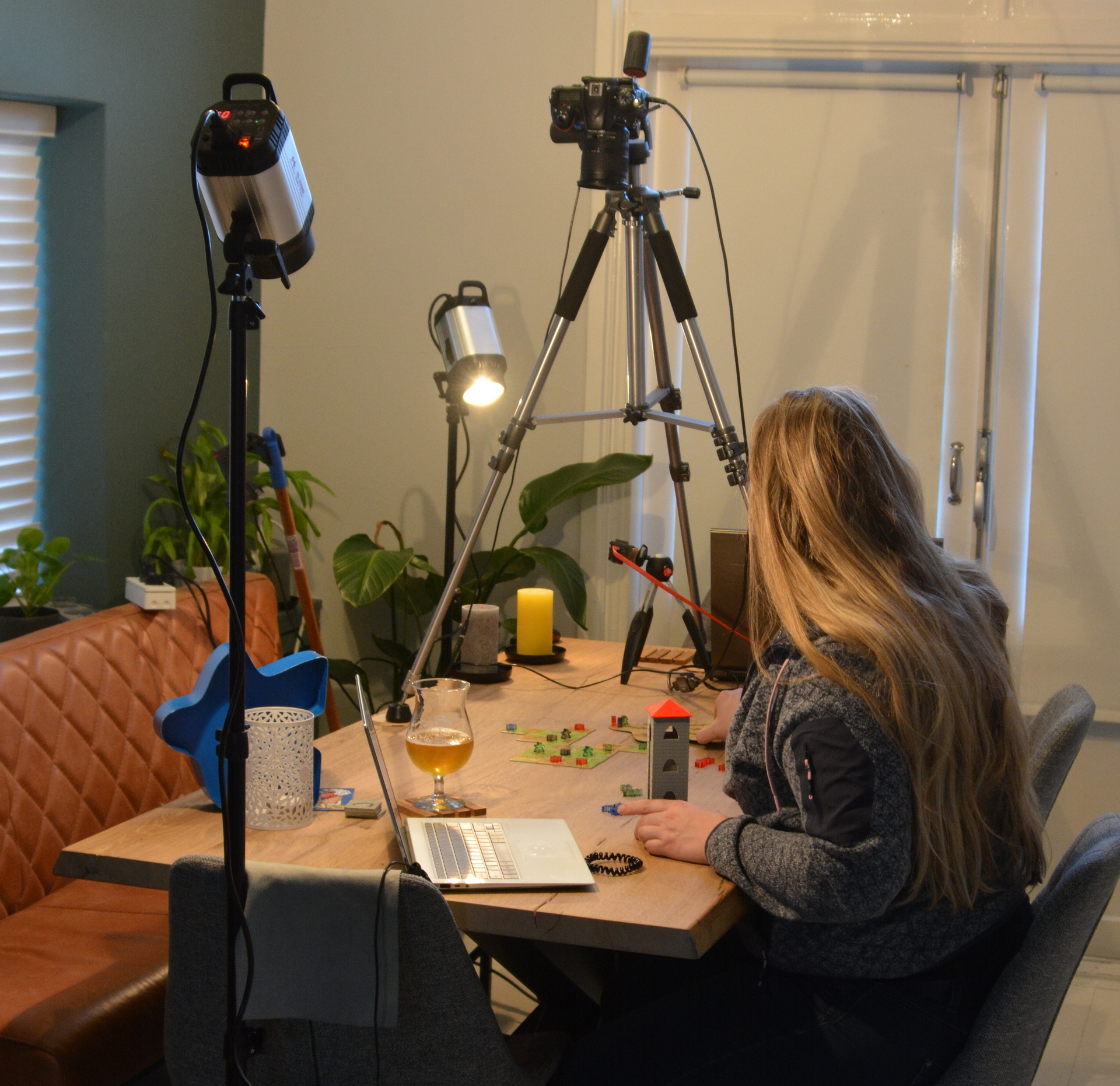
The idea is: Send a url to the players with a streaming camera, you see the game on your screen but you can click on a location on the screen to move a laser pointer to that location.
Below is a proof of concept using the Lasercut worldmap on the wall and a website with a worldmap.
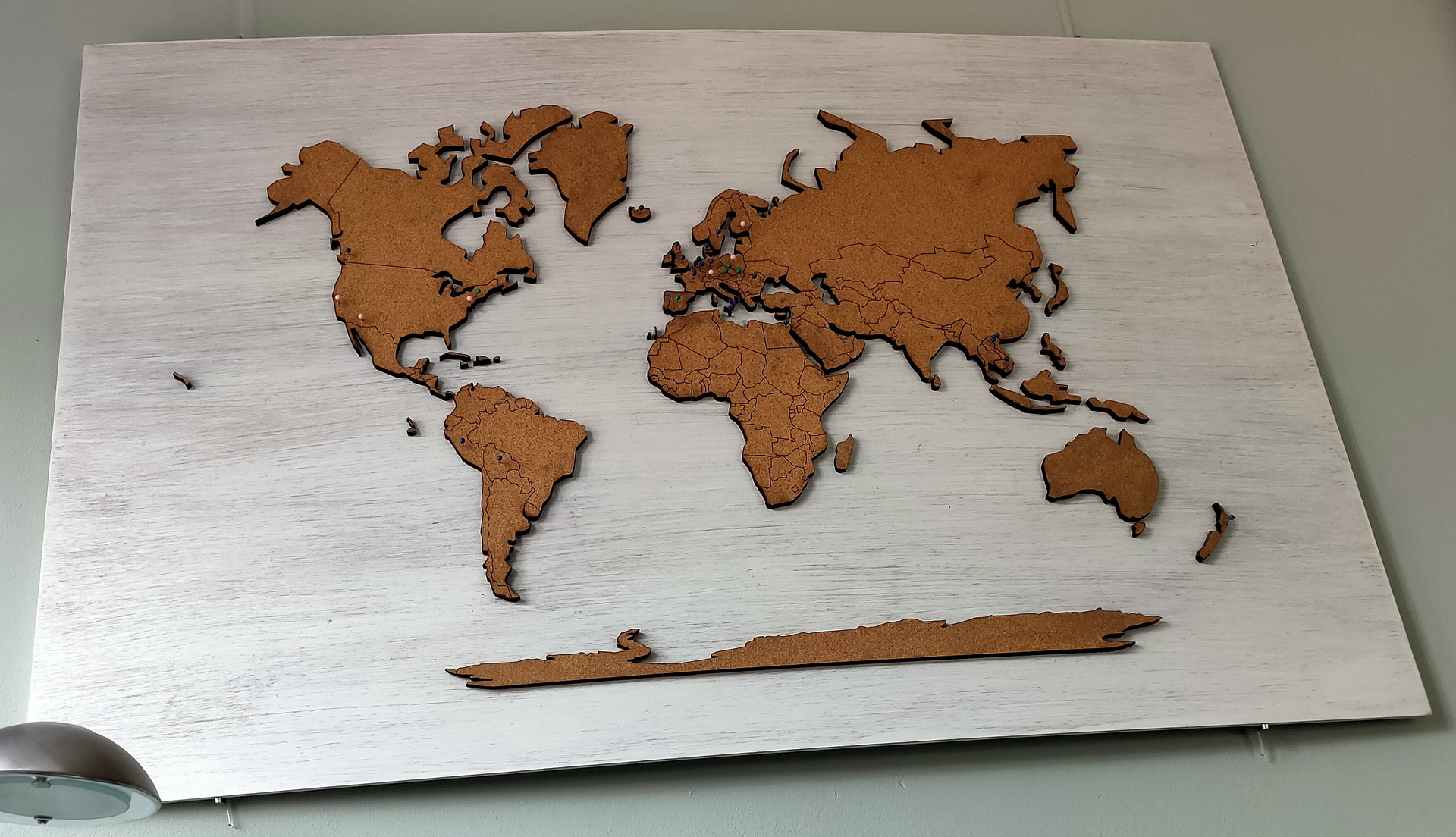
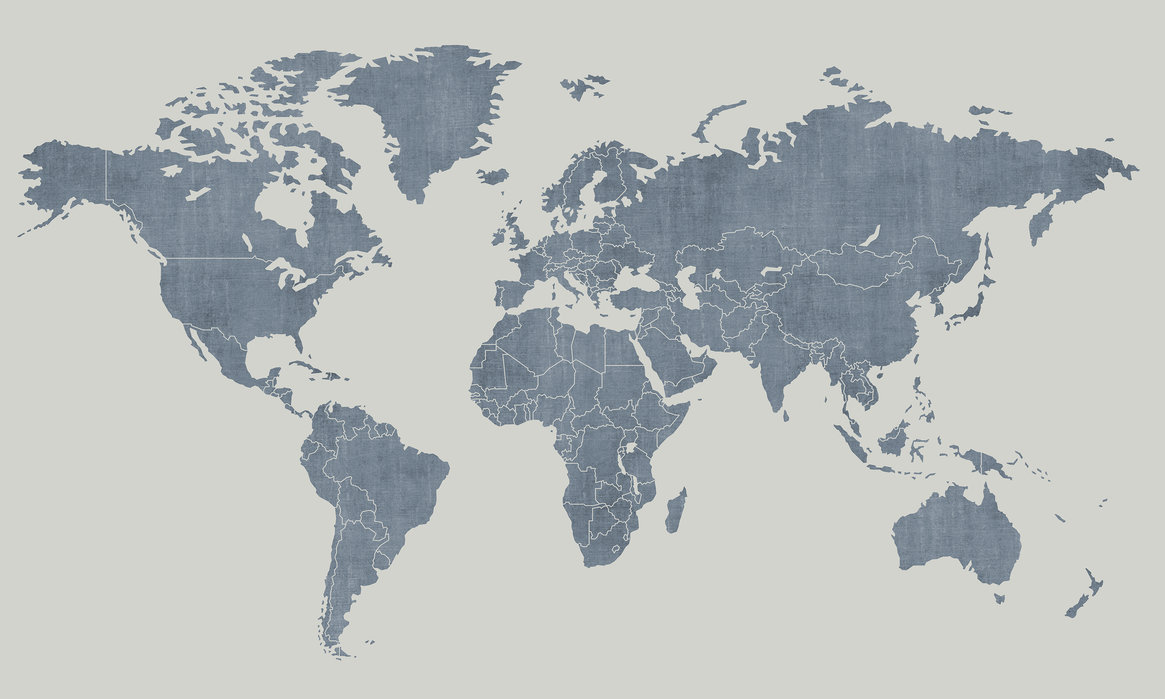
Code to place on the ESP:
This will connect to the MQTT broker and listens for messages on the servo/pan and tilt topic.
#include <ESP8266WiFi.h> #include <PubSubClient.h> #include <Servo.h> Servo pan; Servo tilt; const char* ssid = "MYSSI"; const char* password = "MYWIFIPASS"; const char* mqtt_server = "IPMQTTBROKER"; const char* topic_pan = "servo/pan"; const char* topic_tilt = "servo/tilt"; WiFiClient espClient; PubSubClient client(espClient); long lastMsg = 0; char msg[50]; int value = 0; void setup() { pan.attach(D5); tilt.attach(D6); setup_wifi(); client.setServer(mqtt_server, 1883); client.setCallback(callback); } void setup_wifi() { delay(5); WiFi.begin(ssid, password); while (WiFi.status() != WL_CONNECTED) { delay(500); } } void callback(char* topic, byte* payload, unsigned int length) { String string; for (int i = 0; i < length; i++) { string+=((char)payload[i]); } int pos = string.toInt(); if ( strcmp(topic, topic_pan) == 0 ) { pan.write(pos); } if ( strcmp(topic, topic_tilt) == 0 ) { tilt.write(pos); } delay(15); } void reconnect() { while (!client.connected()) { if (client.connect("ESP8266Client")) { client.subscribe(topic_pan); client.subscribe(topic_tilt); } else { delay(5000); } } } void loop() { if (!client.connected()) { reconnect(); } client.loop(); delay(100); }
Website PHP code:
This has some calibration code to get coordinates lined-up
<?Php $foo_x=$_POST['foo_x']; $foo_y=$_POST['foo_y']; echo "X=$foo_x, Y=$foo_y "; $x=160 - round($foo_x/30); $y=38 - round($foo_y/100); system('/usr/bin/mosquitto_pub -h 10.1.0.17 -t servo/pan -m "' . $x . '"'); system('/usr/bin/mosquitto_pub -h 10.1.0.17 -t servo/tilt -m "' . $y . '"'); ?> <form action='' method=post> <input type="image" alt=' Finding coordinates of an image' src="worldmap.jpg" name="foo" style=cursor:crosshair;/> </form>
POC