Last Updated or created 2023-10-14
Just awesome, nothing else to say.
Just start using it!
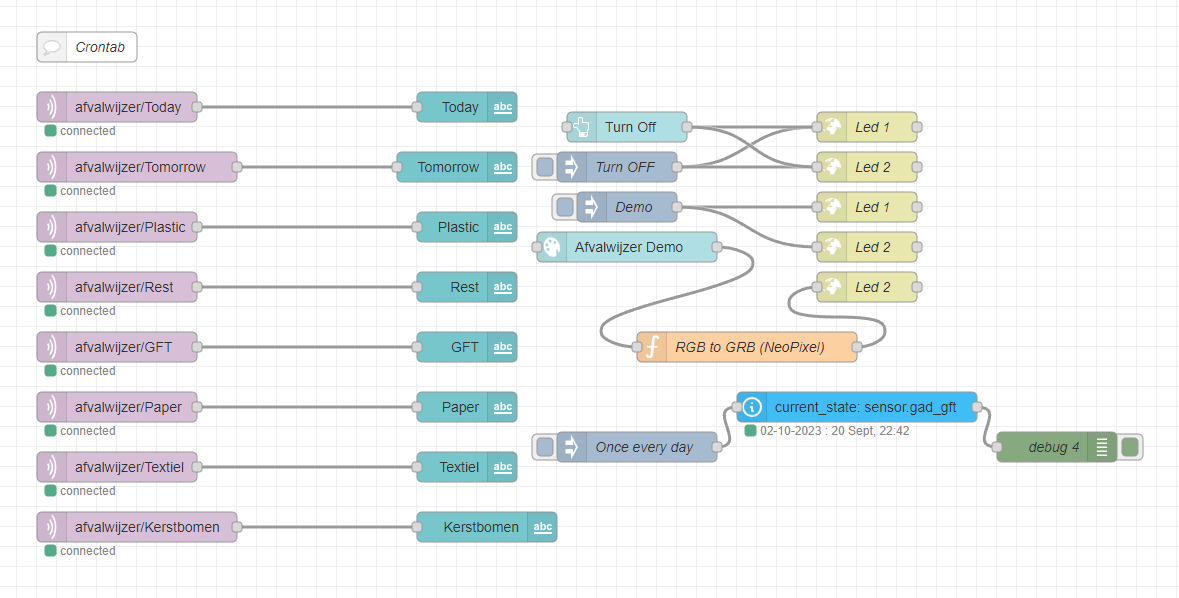
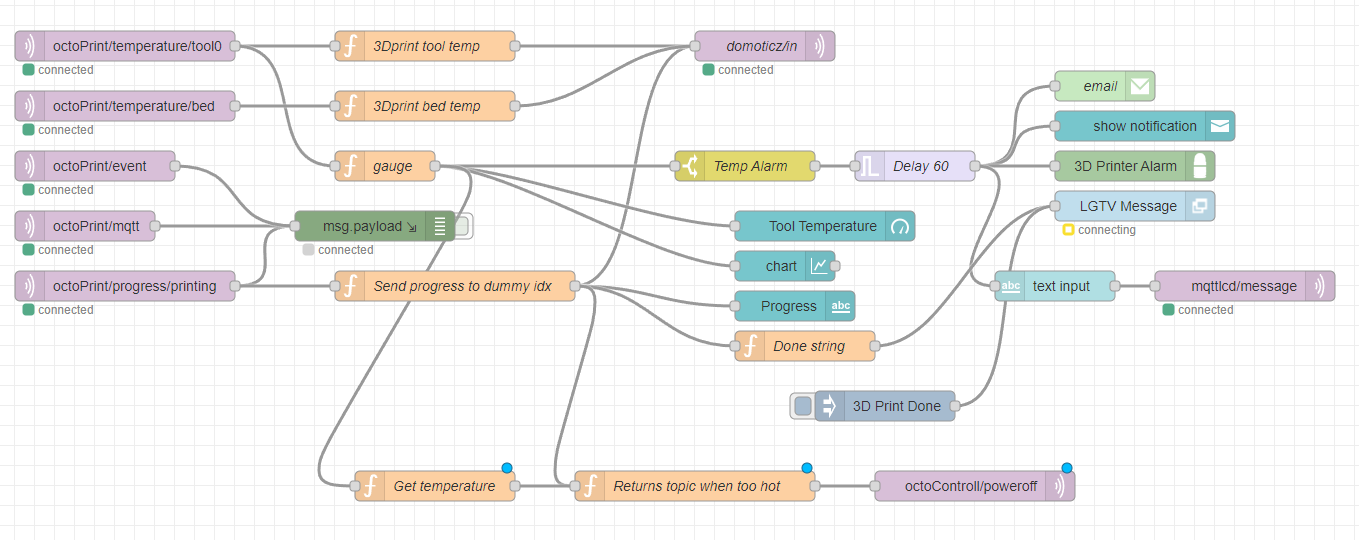
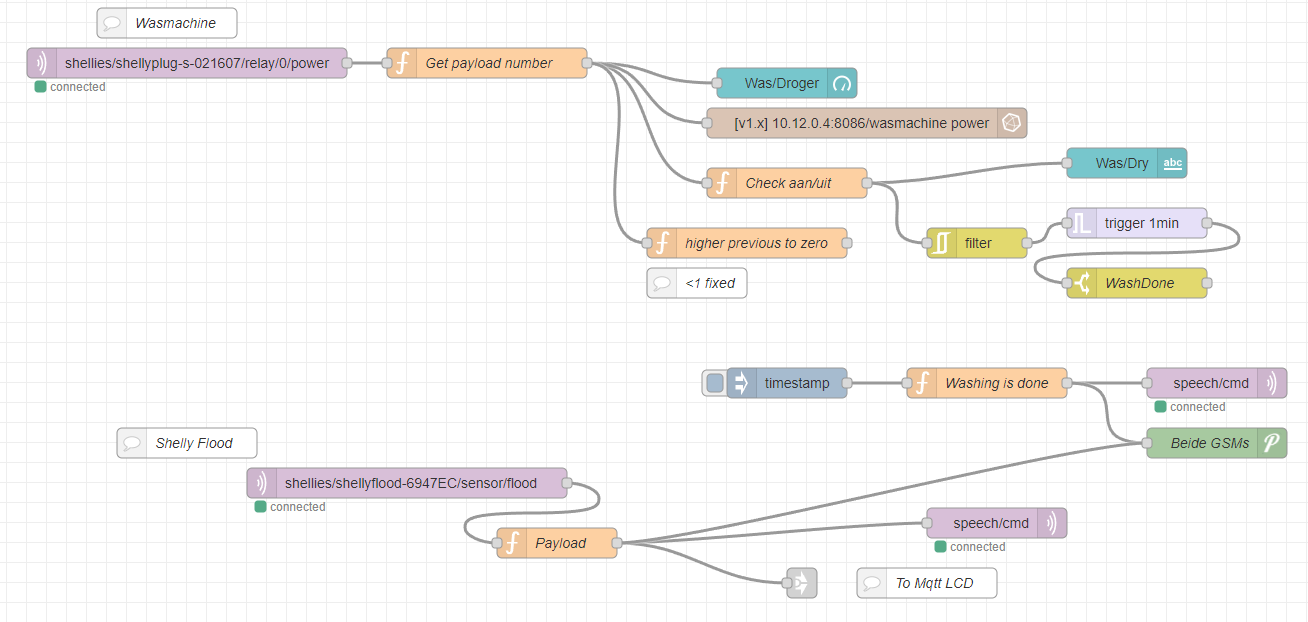
Last Updated or created 2023-10-05
(NOTE, Dosbox can’t cope with the register speed, use real HW or PCem)
Generating a RAW image and Palette, a in a new way
This bash script to convert BMP to Raw and a compiled colorpalette.
(Note: this converts to 8 bit depth, the assembly code in the final assemby program converts to 6 for VGA mode 13h
So this time, i wonΒ΄t have to use the standard VGA palette as mentioned in previous posts.
(Gimp colors > indexed (255 colors) ; save as BMP, exclude colorspace information)
I’m using identify to extract the colorpalette, which i’m converting to DB entries for the fasm compiler
#!/bin/bash if [ $# -lt 1 ] ; then echo "$0 filename" exit 0 fi size=$(stat $1 | grep Size | awk '{ print $2 }') skipsize=$(( $size - 64000)) dd if=$1 of=$1.raw skip=$skipsize bs=1 identify -verbose $1 | awk '/Colormap:/,/Rendering/' | grep -v Colormap | grep -v Rendering | awk '{ print $2 } ' | tr -d '()' | while read ; do echo "db $REPLY" ;done > data.asm fasm data.asm
Code
use16 org 0x100 ; variables CRTC_INDEX = 0x03D4 CRTC_DATA = 0x03D5 INPUT_STATUS = 0x03DA HRETRACE = 0x01 VRETRACE = 0x03 ; bit 3 =8 ? MAXIMUM_SCAN_LINE = 0x09 LINE_OFFSET = 0x13 ; bar upperbar = 1 lowerbar = 399 jmp start ; memory locations for data updown dw 1 direction DB 0 filename DB "firework.raw",0 oldline db 0 start: ; set mode 320x200 256 colors palette mov ah,0x0 mov al,13h int 10h ; clear screen routine, not really needed clearscreen: push ax mov ax, 0a000h mov es, ax pop ax xor di, di inc ax mov cx, 64000 ; 320x200 rep stosb ; set colors ; call file loader call Loadfile call setpalette ; Move loaded file to Screen memory mov ax,0a000h mov es,ax mov ax,6000h mov ds,ax mov si,0 mov di,0 mov cx,320*200/2 rep movsw push cs pop ds ; store org effect2 values mov dx, CRTC_INDEX mov al,LINE_OFFSET out dx,al mov dx, CRTC_DATA in al,dx mov [oldline],al ; after displaying the image or displaying an error, wait for keypress to exit waitforkeyloop: call effect ; Calling the effect MOV AH,1 INT 16h JZ waitforkeyloop XOR AH,AH INT 16h Exit: MOV AX,3 ; default text mode 3 INT 10h MOV AX,4C00h ; exit to dos (terminate process) INT 21h ; loop ends here ; Loads raw 64000 bytes image to screen memory Loadfile: push ds MOV DX,filename MOV AX,3D00h ; open filehandle INT 21h JC Err1 MOV BX,AX ; filehandle MOV CX,64000 mov dx,06000h ; destination 0000:a000h - Screen memory mov ds,dx MOV DX,0 MOV AH,3Fh ; read from file INT 21h JC Err1 MOV AH,3Eh ; close filehandle INT 21h pop ds RET ; print error Err1: push cs ; make ds same as cs pop ds MOV AX,3 ; default text mode 3 INT 10h MOV DX,TxtErr1 ; error MOV AH,09h INT 21h RET effect: cli ; stop interrupts call waitvretrace ; wait for vertical retrace ; gets start scanline and direction mov ax,[updown] mov cl,[direction] cmp cl,0 ; 0 move down jz addcounter dec ax dec ax cmp ax,upperbar ; reached upper bar ? jnz gohere ; jnz mov cl,0 mov [direction],cl jmp gohere addcounter: inc ax inc ax cmp ax,lowerbar ; reached bottom bar? jnz gohere ;jnz mov cl,1 ; change direction mov [direction],cl gohere: mov [updown],ax ; store new location ; al = scanline, call wait for scanline call longwaithretrace ; other effect mov dx, CRTC_INDEX mov al, LINE_OFFSET out dx,al mov dx, CRTC_DATA mov al, 0 out dx,al ; wait scanlines (height of bar) mov ax,400 mov cx,[updown] sub ax,cx call longwaithretrace ; restore effect2 mov dx, CRTC_INDEX mov al, LINE_OFFSET out dx, al mov dx, CRTC_DATA mov al, [oldline] out dx,al sti ; start interrupts again ret ; routine that fixes 8 to 6 bits and sets palette setpalette: ; 8 bits to 6 mov si,coltab mov cx,256*3 rest: mov al,[si] shr al,2 mov [si],al dec cx inc si cmp cx,0 jnz rest ; now set colors mov dx,3c8h xor al,al out dx,al inc dx mov si,coltab mov cx,256*3 rep outsb ret ; this waits for vertical retrace waitvretrace: mov dx,INPUT_STATUS waitv1: in al,dx test al,8 jnz waitv1 waitv2: in al,dx test al,8 jz waitv2 ret ; routine that waits for horizontal retrace waithretrace: mov cl,al mov dx,INPUT_STATUS waith1: in al,dx test al,1 jnz waith1 waith2: in al,dx test al,1 jz waith2 dec cl cmp cl,0 jnz waith1 ret longwaithretrace: mov cx,ax mov dx,INPUT_STATUS lwaith1: in al,dx test al,1 jnz lwaith1 lwaith2: in al,dx test al,1 jz lwaith2 dec cx cmp cx,0 jnz lwaith1 ret TxtErr1 DB "firework.raw not found!",7,10,13,"$" coltab: include 'data.asm'
Last Updated or created 2023-10-05
Not interesting for most of you, but here is the minimal code to display a line using toggling the background color at a specific retrace.
https://www.henriaanstoot.nl/2023/09/12/copperbar-effect-with-image-on-80×86/
3C8h (R/W): DAC Address Write Mode bit 0-7 The color data register (0..255) to be written to 3C9h. Note: After writing the 3 bytes at 3C9h this register will increment, pointing to the next data register. 3C9h (R/W): DAC Data Register bit 0-8? Color value Note: Each read or write of this register will cycle through first the registers for Red, Blue and Green, then increment the appropriate address register, thus the entire palette can be loaded by writing 0 to the DAC Address Write Mode register 3C8h and then writing all 768 bytes of the palette to this register. 3DAh Input Status #1 Register (Read at 3BAh (mono) or 3DAh (color)) 7 6 5 4 3 2 1 0 VRetrace DD VRetrace -- Vertical Retrace "When set to 1, this bit indicates that the display is in a vertical retrace interval.This bit can be programmed, through the Vertical Retrace End register, to generate an interrupt at the start of the vertical retrace." DD -- Display Disabled "When set to 1, this bit indicates a horizontal or vertical retrace interval. This bit is the real-time status of the inverted 'display enable' signal. Programs have used this status bit to restrict screen updates to the inactive display intervals in order to reduce screen flicker. The video subsystem is designed to eliminate this software requirement; screen updates may be made at any time without screen degradation."
Code (fasm)
use16 org 0x100 INPUT_STATUS = 0x03DA start: ; set mode 320x200 256 colors palette mov ah,0x0 mov al,13h int 10h ; press key to exit waitforkeyloop: call effect ; Calling the effect MOV AH,1 INT 16h JZ waitforkeyloop XOR AH,AH INT 16h Exit: MOV AX,3 ; default text mode 3 INT 10h MOV AX,4C00h ; exit to dos (terminate process) INT 21h effect: cli ; stop interrupts call waitvretrace ; wait for vertical retrace mov al, 0 ; set color index 0 to black (needs to be converted to a function mov dx, 3c8h out dx, al inc dx ; now 3c9h mov al, 0h out dx, al ; set R = 0 mov al, 0h out dx, al ; set G = 0 mov al, 0h out dx, al ; set B = 0 mov al,30h ; al = scanline, call wait for scanline call waithretrace mov al, 0 ; set color index 0 to white mov dx, 3c8h out dx, al inc dx mov al, 255 out dx, al mov al, 255 out dx, al mov al, 255 out dx, al ; wait 1 scanlines (height of bar) mov al,1h call waithretrace ; draw black again mov al, 0 ; set color index 0's rgb value mov dx, 3c8h out dx, al inc dx ; now 3c9h mov al, 0 out dx, al out dx, al out dx, al sti ; start interrupts again ret ; this waits for vertical retrace waitvretrace: mov dx,INPUT_STATUS waitv1: in al,dx test al,8 jnz waitv1 waitv2: in al,dx test al,8 jz waitv2 ret ; routine that waits for horizontal retrace ; al sets number of retraces waithretrace: mov cl,al mov dx,INPUT_STATUS waith1: in al,dx test al,1 jnz waith1 waith2: in al,dx test al,1 jz waith2 dec cl cmp cl,0 jnz waith1 ret
My bash file to copy com file to floppy image to use in PCem.
PCem right button disk change drive A:
fasm one-line.asm # disk.img is een msdos boot floppy image sudo mount -o loop disk.img mountpoint sudo cp *com mountpoint/ sudo cp *bmp mountpoint/ sudo umount mountpoint
Last Updated or created 2023-10-05
Yesterday I got my MAX9814 in, last night I got it working.
Used the leds from a lightpainter project to test controlling the leds.
Why is it, that it doesn’t matter how much components and ledstrips you buy, you alway need more.
Arduino Nano, using FastLeds library and FFT.
Ledstrip is WS2812, and the MAX8914 microphone
Last Updated or created 2023-10-05
While watching a online python course, I was writing the code for a music guessing game (Highland Bagpipe Tunes)
The core is working, now it’s a matter of filling this “pruts” with tunes.
Switching between python, php, bash and C is a nightmare π
Then the postman came .. with goodies.
I needed the MAX9814 analog microphone with amplifier, all of my other sensors were not up to the task.
So I switched to this WIP with the MAX9814.
I want to make a little gadget using an Arduino and 9 leds, which uses FFT to blink which note I am playing on my Highland Pipes.
So detecting is working, now I have to attach a bunch of leds.
Next thing I did today:
Getting my waveshare RP-2040 Zero working with micropython.
Great the little NeoPixel Led on the board.
Steps to get this working:
Want to run program @boot ?
save -> to device, and call main.py
Last Updated or created 2023-10-05
Plays RAW adlib songs in 100 lines of code … kindda
Using information from here:
https://moddingwiki.shikadi.net/wiki/RAW_Format_(Adlib)
And using fasm to compile I can play captured raw songs.
But something is still off ?!?
It sounds a little different, and I need to implement a better timer routine. (Below my version and opencubicplayer)
This is a test for my bootloader, playing music from my bootblock!
CODE
use16 org 0x100 ctrlreg=0388h datareg=0389h mainloop: ; set speed (Byte 8,9 from the raw file) mov ax,[tune+8] mov [clockspeed],ax ; call player call rawreg ; wait 0.5 sec for exit mov bx,6 call waitmore jmp exit rawreg: mov bx,tune+0ah ; start of song at offset ah ; order registerdata, register! ; Are there more control codes? ??? lraw: mov cx,[bx] ; reg = 2 - check data cmp ch,2 je checkreg2 ; reg = 0 - cyclewait cmp ch,0 je cyclewait ; data = FFFF - end song - end play routine cmp cx,0ffffh jne skipr ret cyclewait: ; waits cl times waitroutine cylloop: call waitlong dec cl jnz cylloop inc bx inc bx jmp lraw checkreg2: ; check low opl cmp cl,1 jne checkh mov ch,0 mov [highlow],ch jmp incandret checkh: ; check high opl cmp cl,2 jne check00 mov ch,1 mov [highlow],ch jmp incandret check00: ; set new speed cmp cl,0 jne incandret inc bx inc bx mov ax,[bx] mov [clockspeed],ax incandret: ; next double byte in the song please inc bx inc bx jmp lraw skipr: ; sends data to the control and data registers mov dx,ctrlreg mov al,[highlow] cmp al,0 je regokay inc dx inc dx regokay: mov al,ch out dx,al ; call waitshort ; not needed for newer adlib cards mov dx,datareg mov al,[highlow] cmp al,0 je regokay2 inc dx inc dx regokay2: mov al,cl out dx,al call waitlong inc bx inc bx jmp lraw waitshort: push ax push cx push dx mov cx, 0 ;HIGH WORD. mov dx, 010h ;LOW WORD. mov ah, 86h ;WAIT. int 15h pop dx pop cx pop ax ret waitlong: push bx push ax push cx push dx mov cx, 0 ;HIGH WORD. mov dx, [clockspeed] shr dx,1 mov ah, 86h ;WAIT. int 15h pop dx pop cx pop ax pop bx ret waitmore: ; in bx == 12h is 1 sec ; destroys ax,bx,cx,dx push ax push bx push cx push dx mov ax,0h int 1ah add dx, bx mov bx,dx waitloop: mov ax,0h int 1ah cmp bx,dx jnz waitloop pop dx pop cx pop bx pop ax ret exit: mov dx,0388h mov al,0b0h out dx,al inc dx xor al,al out dx,al mov ax,04c00h int 21h clockspeed: dw 0 highlow: db 0 tune: file 'RAWSONG.RAW'
Last Updated or created 2023-10-05
Loading a 320×200 image from 14 cilinders. There is no msdos on the floppy!
9 sectors, 14 cilinders, 1 head * sector size (512 bytes) = 64512 bytes
Mode 13h (320×200 265 colors)
Cuting the raw part from a BMP (see previous post)
root@battlestation:/mnt/# ls -la MAD.bmp -rw-rw-r-- 1 fash fash 65078 Sep 14 15:57 MAD.bmp I need 64000 bytes (320x200) 65078-64000 = 1078 root@battlestation:/mnt/# dd if=MAD.bmp of=mad.raw skip=1078 bs=1 64000+0 records in 64000+0 records out 64000 bytes (64 kB, 62 KiB) copied, 0.441618 s, 145 kB/s
I use debug to write to the sectors
debug mad.raw -w100 0 9 7f (write from address 100 drive=0 startsector=9 (cylinder 1) and 7f sectors long
Wrote a little sector viewer to debug/view data written.
I will post the code after some code cleaning and adding some comments
Last Updated or created 2023-10-05
I’m still having problems getting a working floppy drive in my machine.
(Broken FDD card, drive errors etc)
The raster bar (also referred to as rasterbar or copperbar) is an effect used in demos and older video games that displays animated bars of colour, usually horizontal, which additionally might extend into the border, a.k.a. the otherwise unalterable area (assuming no overscan) of the display
I first tried to get it working in DosBOX, but thats a mess.
Good for simple emulation but not hardcore register manipulation.
Below dosbox
Three examples below are in PCem
use16 org 0x100 CRTC_INDEX = 0x03D4 CRTC_DATA = 0x03D5 INPUT_STATUS = 0x03DA MAXIMUM_SCAN_LINE = 0x09 LINE_OFFSET = 0x13 jmp start updown DB 30 direction DB 0 filename DB "shoes.bmp",0 start: ; set mode 320x200 256 colors palette mov ah,0x0 mov al,13h int 10h ; clear screen routine, not really needed clearscreen: push ax mov ax, 0a000h mov es, ax pop ax xor di, di inc ax mov cx, 64000 ; 320x200 rep stosb ; call file loader call Loadfile push cs pop ds ; after displaying the image or displaying an error, wait for keypress to exit waitforkeyloop: call effect ; Calling the effect MOV AH,1 INT 16h JZ waitforkeyloop XOR AH,AH INT 16h Exit: MOV AX,3 ; default text mode 3 INT 10h MOV AX,4C00h ; exit to dos (terminate process) INT 21h Loadfile: MOV DX,filename MOV AX,3D00h ; open filehandle INT 21h JC Err1 MOV BX,AX ; filehandle MOV CX,0FFFFh ; size mov dx,0a000h ; destination 0000:a000h - Screen memory mov ds,dx MOV DX,0 MOV AH,3Fh ; read from file INT 21h JC Err1 MOV AH,3Eh ; close filehandle INT 21h RET ; print error Err1: push cs ; make ds same as cs pop ds MOV DX,TxtErr1 ; error MOV AH,09h INT 21h RET effect: cli ; stop interrupts call waitvretrace ; wait for vertical retrace mov al, 0 ; set color index 0 to black (needs to be converted to a function mov dx, 3c8h out dx, al inc dx ; now 3c9h mov al, 0h out dx, al ; set R = 0 mov al, 0h out dx, al ; set G = 0 mov al, 0h out dx, al ; set B = 0 ; gets start scanline and direction mov al,[updown] mov ah,[direction] cmp ah,0 jz addcounter dec al cmp al,30 jnz gohere mov ah,0 mov [direction],ah jmp gohere addcounter: inc al cmp al,100 jnz gohere mov ah,1 mov [direction],ah gohere: mov [updown],al ; al = scanline, call wait for scanline call waithretrace mov al, 0 ; set color index 0 to blueish mov dx, 3c8h out dx, al inc dx mov al, 11h out dx, al mov al, 22h out dx, al mov al, 33h out dx, al ; wait 10 scanlines (height of bar) mov al,10 call waithretrace ; draw black again mov al, 0 ; set color index 0's rgb value mov dx, 3c8h out dx, al inc dx ; now 3c9h mov al, 0 out dx, al ; set R = 11h mov al, 0h out dx, al ; set G = 22h mov al, 0h out dx, al ; set B = 33h sti ; start interrupts again ret ; this waits for vertical retrace waitvretrace: mov dx,INPUT_STATUS waitv1: in al,dx test al,8 jnz waitv1 waitv2: in al,dx test al,8 jz waitv2 ret ; routine that waits for horizontal retrace waithretrace: mov cl,al mov dx,INPUT_STATUS waith1: in al,dx test al,1 jnz waith1 waith2: in al,dx test al,1 jz waith2 dec cl cmp cl,0 jnz waith1 ret TxtErr1 DB "shoes.bmp not found!",7,10,13,"$"
Last Updated or created 2023-10-05
This is a work in progress, below are my Lab notes.
I want to rewrite pieces we made for a demo, loading images and effects from a floppydisk bootloader.
Without looking at old code (which was written using Masm), I wanted to learn the steps using Fasm.
I started with a boot sector program, It should do the following.
I got it working, half that is.
In the past I used real disks, and now a virtual disk, maybe thats the difference?
First Code
use16 org 0x7c00 mov ah,0x0 mov al,0x13 int 10h mov ax, 0a000h mov es, ax xor di, di mov ax, 50 mov cx, 64000 rep stosb loophere: jmp loophere times 510 - ($-$$) db 0 dw 0xaa55
Compiling and starting:
fasm mybootblock.asm qemu-system-x86_64 --drive format=raw,file=mybootblock.bin
This works, it sets the graphical mode and clears the screen.
Second Code
Skipping the int 25h version
## Track read part xor ax, ax ; DS = 0 mov ds, ax cld mov ah, 2h ; int13h function 2 track read mov al, 2 ; number of tracks ( should be 2 for reading only palette) mov ch, 0 ; from cylinder number 0 mov cl, 2 ; the sector number 2 - second sector (starts from 1, not 0) mov dh, 0 ; head number 0 xor bx, bx ; BX = 0 mov es, bx ; ES = 0 mov bx, 7e00h ; Offset from above int 13h call setpal ## End part with setpalette routine ## appending palette.colors ## and a raw image setpal: mov dx,3c8h xor al,al mov di, ax out dx,al inc dx mov cx,256*3 mov si,07e00h rep outsb ret times 510 - ($-$$) db 0 dw 0xaa55 include 'palette.colors' times 2048 - ($-$$) db 0 file 'image.raw'
Seems there is still a header on the RAW file, lets look at how I made this.
NOTE! .. Below converts an image with a STANDARD VGA palette, not a custom one as used above
Looking with ghex at the file I saw that there was a header 0x415 bytes large.
(Probably still palette colors in there)
dd if=shoes.bmp of=cutshoe.bmp bs=1 skip=1078 (0x415h + 3?)
worked for me
Loading the extra tracks didn’t work for me?!?!
But how could I define tracks/sectors and heads on a virtual floppy?
I tried to write sectors using debug.com
start dosbox imgmount a: /tmp/floppy.img -t floppy debug.com bootsector.bin -r bx 01 -r cx 512 ; set bx:cx for size -w 100 0 0 1 ; write from address 100, drive 0 (a), sector 0, number of sectors ; testing -l 100 0 0 1 ;load sector to addr 100 drive 0 sector 0 number of sectors This used to work with real disks on a real machine, not in dosbox ?!?! my way to create a disk in linux dd if=bootblock.bin of=disk1.img bs=512 count=1 seek=0 dd if=palette.col of=disk1.img bs=512 count=1 seek=1 # or 2? dd if=shoes.raw of=disk1.img bs=512 count=10000 seek=17
It looks like I can’t read futher than 18 sectors on a virtual floppy.
What next? Head=1? Cylinder=1?
Below the info from a floppy image before altering.
DOS/MBR boot sector, code offset 0x3c+2, OEM-ID “MSDOS5.0”, root entries 224, sectors 2880 (volumes <=32 MB), sectors/FAT 9, sectors/track 18, serial number 0x1c2a0d0f, unlabeled, FAT (12 bit), followed by FAT
Appending the RAW to a executable gave me problems to.
(Without making a bootdisk)
Above and below weird data. Appending the data to the executable needs some work also.
At least for today let me display this image correctly π
use16 org 0x100 ; set mode 320x200 256 colors palette mov ah,0x0 mov al,13h int 10h ; clear screen routine, not really needed clearscreen: push ax mov ax, 0a000h mov es, ax pop ax xor di, di inc ax mov cx, 64000 ; 320x200 rep stosb ; call file loader call Loadfile ; after displaying the image or displaying an error, wait for keypress to exit waitforkeyloop: MOV AH,1 INT 16h JZ waitforkeyloop XOR AH,AH INT 16h Exit: MOV AX,3 ; default text mode 3 INT 10h MOV AX,4C00h ; exit to dos (terminate process) INT 21h Loadfile: MOV DX,filename MOV AX,3D00h ; open filehandle INT 21h JC Err1 MOV BX,AX ; filehandle MOV CX,0FFFFh ; size mov dx,0a000h ; destination 0000:a000h - Screen memory mov ds,dx MOV DX,0 MOV AH,3Fh ; read from file INT 21h JC Err1 MOV AH,3Eh ; close filehandle INT 21h RET ; print error Err1: push cs ; make ds same as cs pop ds MOV DX,TxtErr1 ; error MOV AH,09h INT 21h RET filename DB "shoes.bmp",0 TxtErr1 DB "shoes.bmp not found!",7,10,13,"$"
Tomorrow .. back to the track loader
Last Updated or created 2023-10-05
Quiet days, I working on some art.
But here are the last ‘prutsen’
My current Wifi setup
I’ve got a Wifi outside of my network for guest and emergency. ( 2 SSIDs)
Then a main Wifi router in my livingroom, one in my workshop/studio and one in the Attic (Electronics Lab)
So three main Wifi AccessPoints. These all have the same SSID’s but on different frequencies. That way i’ve got roaming in and outside my house.
Also some virtual accesspoints are configured.
I’ve got a main, folkband, IOT, guest-inside all on 2.4Ghz and 5Ghz.
I watched a lot of YT presentations about Mikrotik Wifi.
So I ended up with DFS safe channels 20Mhz for 2.4 and 20/40Mhz Ce for 5Ghz. (subchannels for each after some frequency scanning)
(2.4 does a failback to 20Mhz whenever there is even one client detected which connects only on this band. Such as some old IOT stuff)
2.4 in only 1,6 and 11 no overlap, each on another device.
300Mbps is sufficient for my wifi π
I’ve got accesslists in place and i’m going to read into kicking a client when the signal strenght is below -50dB
80386 (DX) Computer
Besides my 8088 and 8086 machines I needed a machine which could run our old demo’s. So I bought a new toy.
It has 8Mb Ram and runs at 40Mhz.
I’ve noticed that many of my VGA register manipulation code, can’t be run on a modern VGA monitor, I need to use a CRT for that .. Another thing to buy
Lilygo T-Display S3 Streaming
Not my code: https://github.com/Steve5451/esp32-stream-desktop
A very cool project!
Needed to fix arduino code, due to the TFT_eSPI library issues.
And I’ve got a S3 with another resolution, but that was an easy fix.
Then needed to reinstall nodejs with another version.
Had to modify the code because the tcp server would not start.
Weird errors logging, but in the end fixed … very cool
I probably end up designing a 3D printed case that looks like a monitor or tv.