Last Updated or created 2022-11-08
Making red wine from the grapes we got from our neighbours.
Funny movie – feet i a bucket of wine
Some books about the subject we own
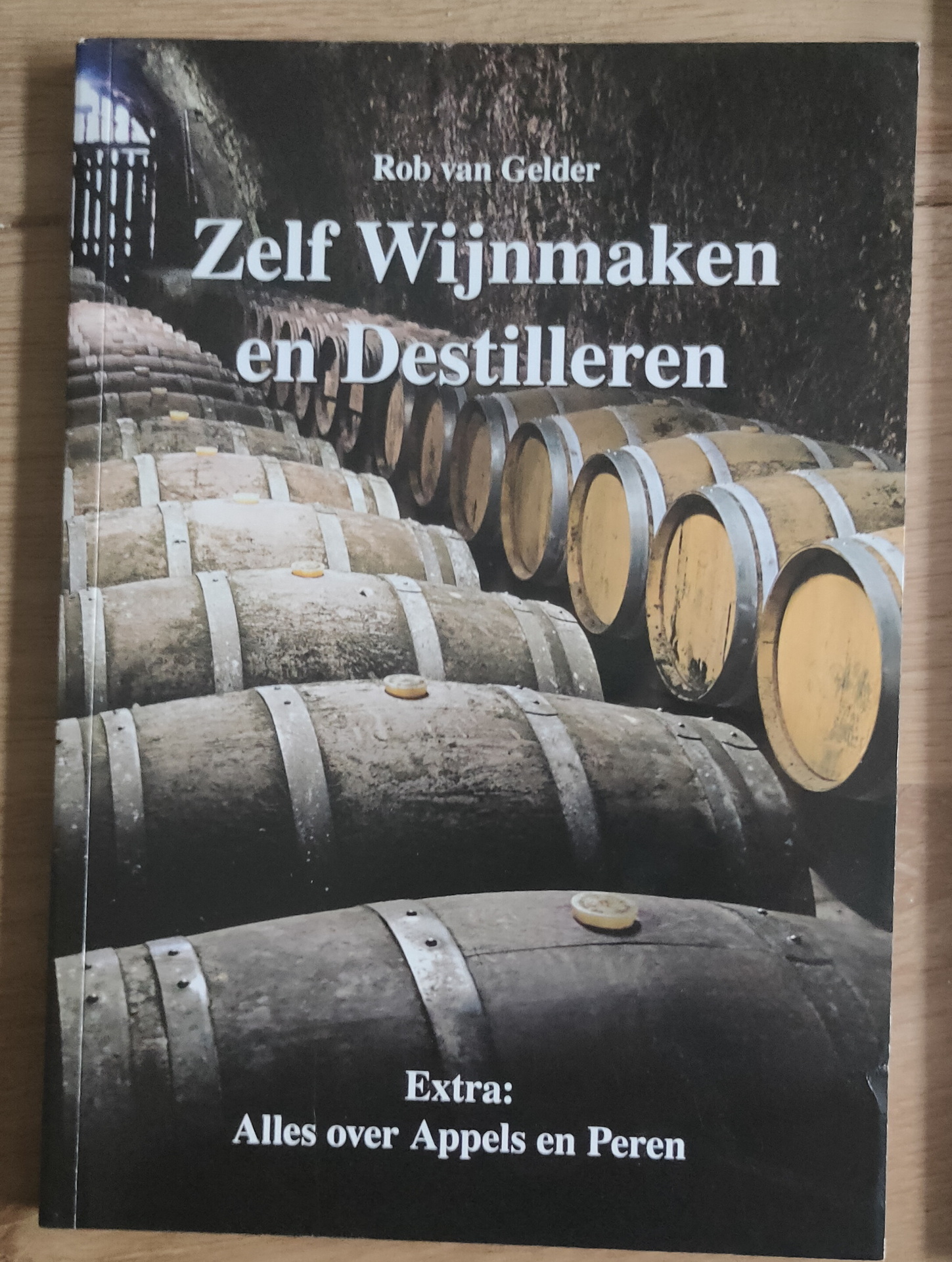
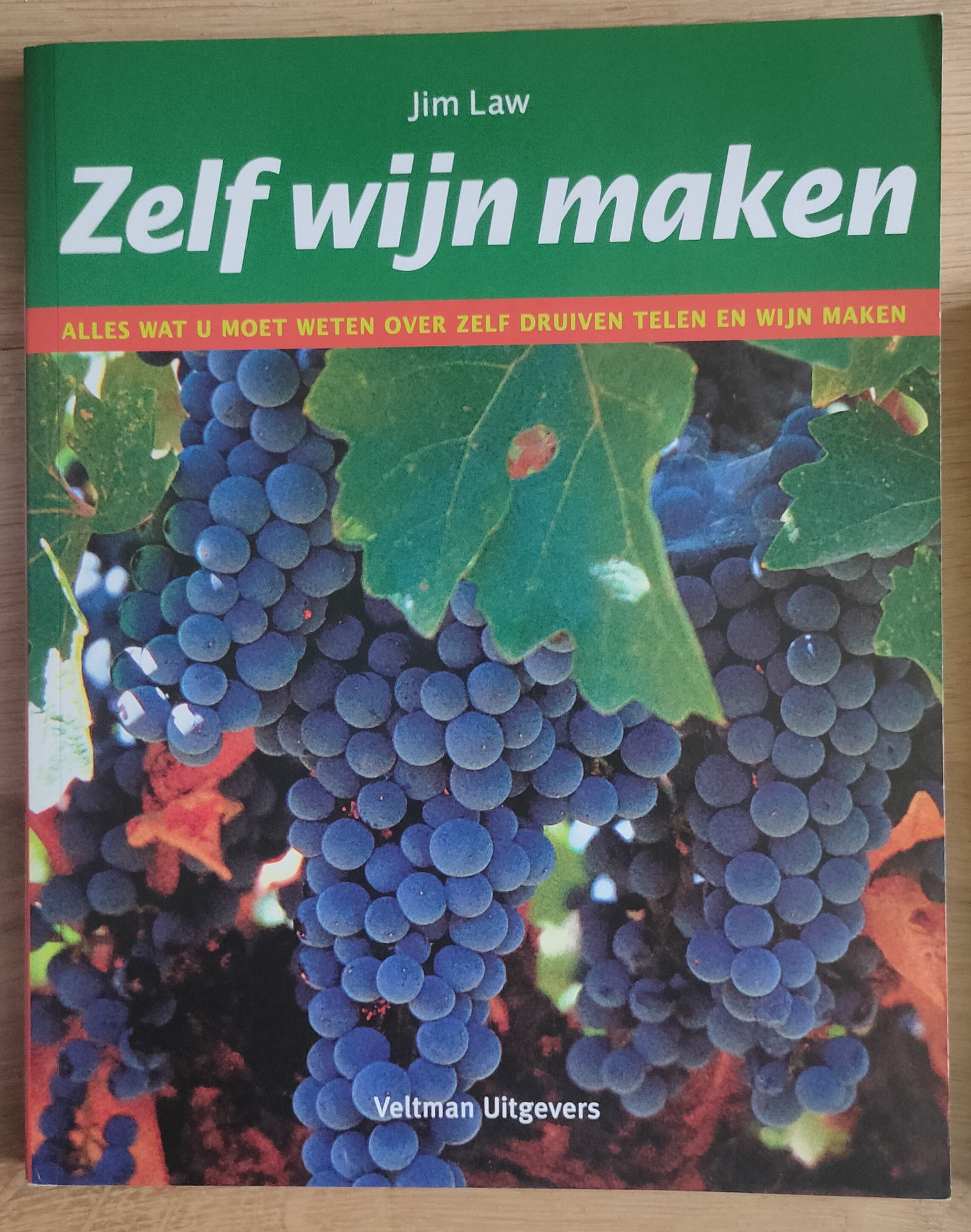
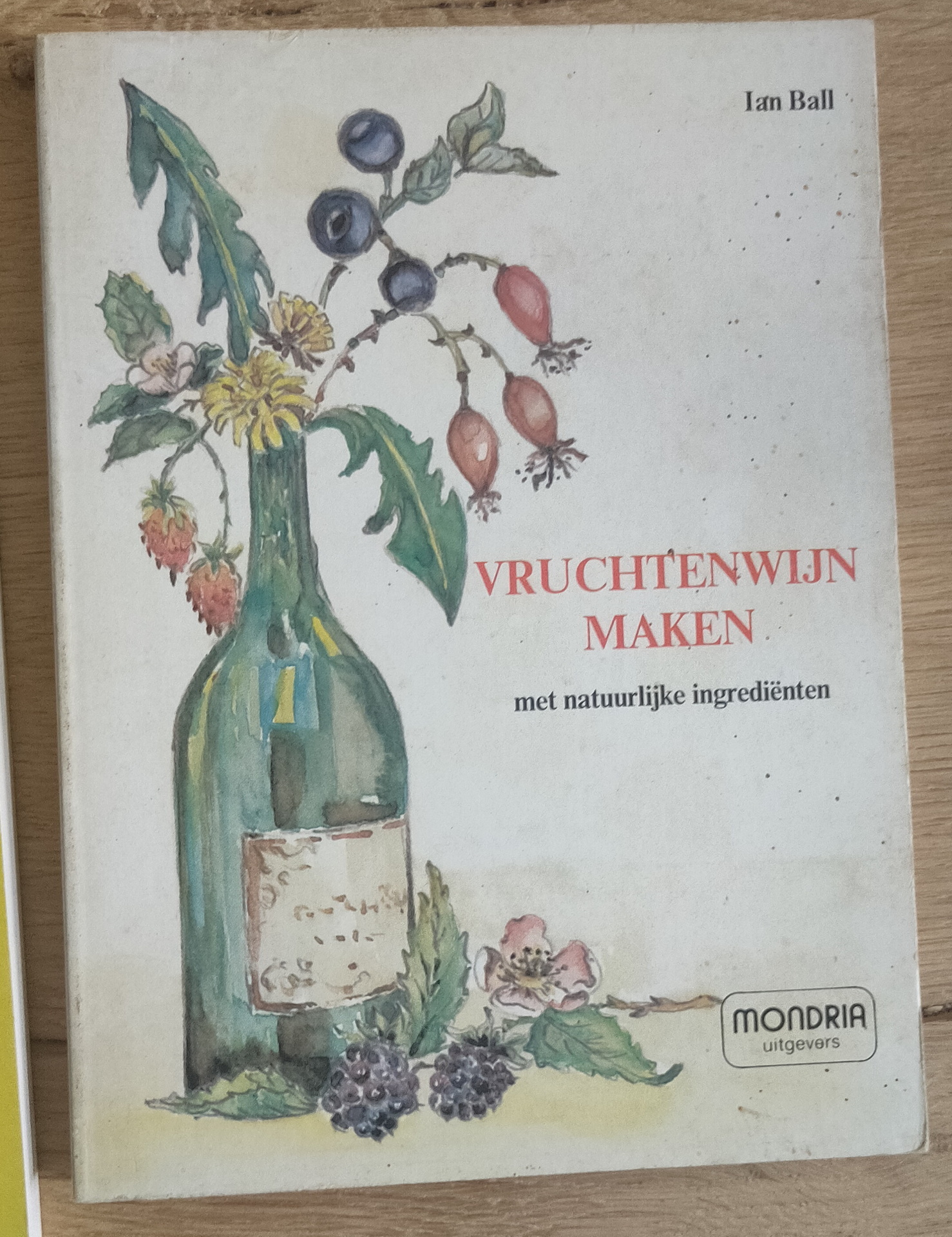
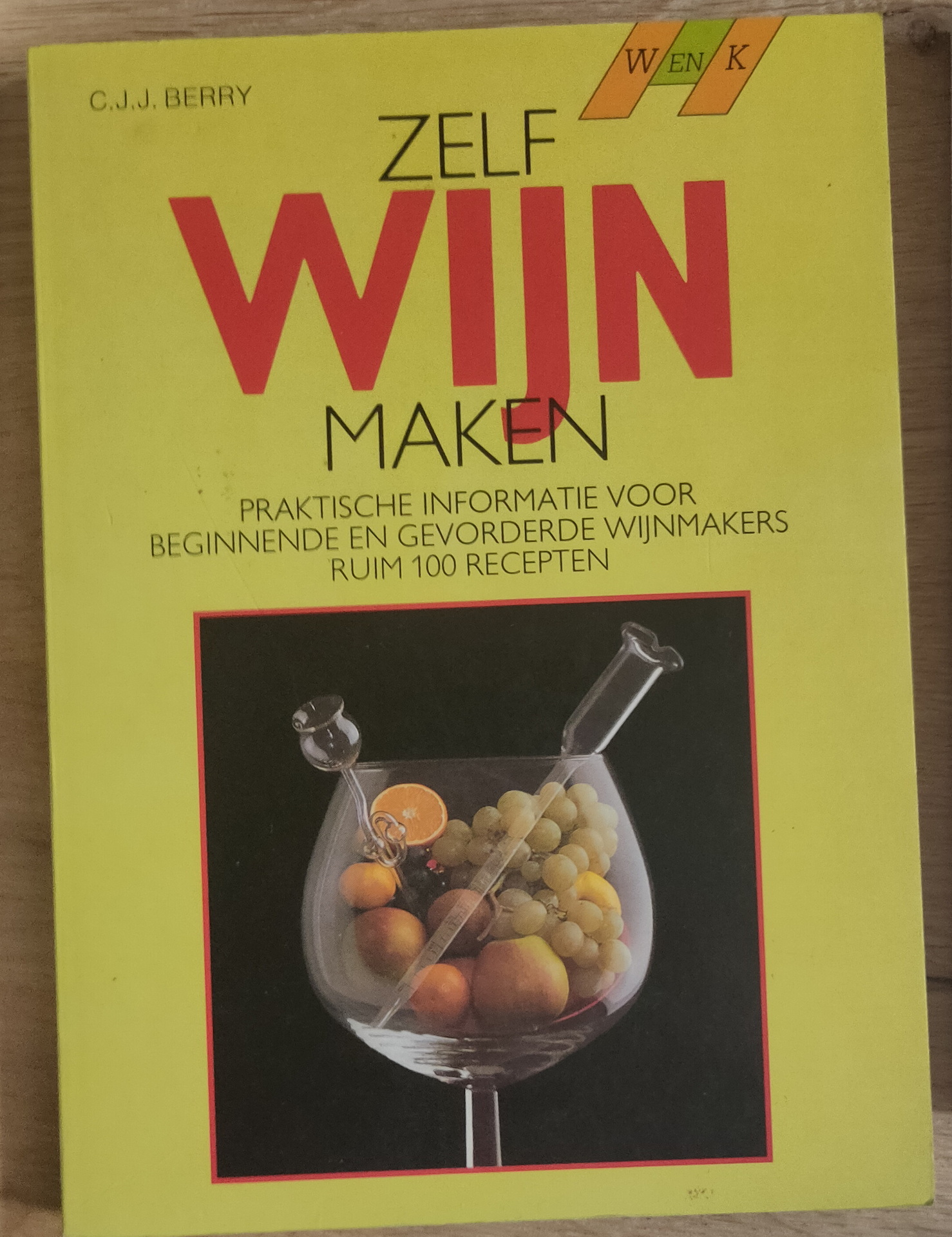
Last Updated or created 2022-11-08
Last Updated or created 2023-01-03
A script to check the age of sensors.
Sometimes you don’t have a good 433Mhz connection.
Or the battery has died of your sensor.
This script will warn you.
sensoragescript.sh (for cron)
#!/bin/bash #Call as follows #sensoragescript.sh <ipdomoticz> <idx-of-sensor> <age-to-test-in-seconds> now=$(date +%s) lastupdate=$(curl -s -i -H "Accept: application/json" "http://$1:8080/json.htm?type=devices&rid=$2" | grep LastUpdate | cut -f4 -d\" ) #echo $lastupdate seen=$(date -d "$lastupdate" +%s) #echo $seen #echo "$(( $now - $seen))" difftime="$(( $now - $seen))" if [ $difftime -gt $3 ] ; then echo "WARN : too old - $difftime seconds" exit 1 else echo "OK : $difftime seconds" exit 0 fi
Outputs:
./new.sh 192.168.1.1 123 60 OK : 22 seconds ./new.sh 192.168.1.1 123 60 WARN : too old - 69 seconds
check_mk / icinga check
#!/bin/bash IPDOMO=192.168.1.1 IDX=123 agewarn=300 now=$(date +%s) lastupdate=$(curl -s -i -H "Accept: application/json" "http://$IPDOMO:8080/json.htm?type=devices&rid=$IDX" | grep LastUpdate | cut -f4 -d\" ) seen=$(date -d "$lastupdate" +%s) difftime="$(( $now - $seen))" if [ $difftime -gt $agewarn ] ; then echo "1 \"WARN - Age check Fridge\" realage=$difftime|age=$agewarn Age of fridge" exit 1 else echo "0 \"OK - Age check Fridge\" realage=$difftime|age=$agewarn Age of fridge" exit 0 fi
Last Updated or created 2023-01-03
For my home automation i’m using Home Assistant and Domoticz.
All 433Mhz Temperature/Humidity are connected to a RFXcom device on two domoticx instances. (Master slave construction)
I’ve made a php script and a bash script to draw all sensors on a floorplan in realtime.
There is also a cron running which takes a snapshot of the generated image every 5 minutes.
These images are being converted to MP4 and animated GIF to have a timelapse with all temperatures displayed on a floorplan.
The circles are where sensors are placed.
Colors are from blue till red, representing the heat.
In the center is the measured temperature value.
The (shortened) PHP script: (index.php)
<?php header('Content-type: image/png'); // This is the floorplan empty .. $png_image = imagecreatefrompng('plattegrondenmerge.png'); $white = ImageColorAllocate($png_image, 0, 0, 0); $max = 40; $min = -10; // living // getstate is a bash script (see below which gets the values from domoticz using curl) // 840 is the domoticz idx $temp840 = shell_exec('./getstate 840'); // A gray circle will be drawn if the temperature age is > 500 seconds $age = shell_exec('./new.sh 18 840 500 >/dev/null || echo gray'); // location of circle $start_x = 950; $start_y = 760; $line = $temp840 + 10; // get x-th line from colors $colorfromlist = shell_exec("tail --lines=$line ./colors2 | head -1"); if(strpos($age, "gray") !== false){ $colorfromlist = "128,128,128"; }; $colors = explode(",", $colorfromlist); $color = imagecolorallocatealpha($png_image, $colors[0], $colors[1], $colors[2], 50); // draw circle imagefilledellipse ($png_image, $start_x, $start_y, 175, 175, $color); $start_x = $start_x - 70; $start_y = $start_y + 15; // add text imagettftext($png_image, 24, 0, $start_x, $start_y, $white, './verdana.ttf', $temp840); // winecellar $temp840 = shell_exec('./getstate 839'); $age = shell_exec('./new.sh 18 839 700 >/dev/null || echo gray'); $start_x = 560; $start_y = 840; $line = $temp840 + 10; $colorfromlist = shell_exec("tail --lines=$line ./colors2 | head -1"); if(strpos($age, "gray") !== false){ $colorfromlist = "128,128,128"; }; $colors = explode(",", $colorfromlist); $color = imagecolorallocatealpha($png_image, $colors[0], $colors[1], $colors[2], 50); imagefilledellipse ($png_image, $start_x, $start_y, 175, 175, $color); $start_x = $start_x - 70; $start_y = $start_y + 15; imagettftext($png_image, 24, 0, $start_x, $start_y, $white, './verdana.ttf', $temp840); // ETC ETC imagesavealpha($png_image, TRUE); imagepng($png_image); imagedestroy($png_image); ?>
getstate bash script
(gets the temperature from domoticz instance1 given an idx)
#!/bin/bash curl -s --connect-timeout 2 --max-time 5 "http://ip-domoticz1:8080/json.htm?type=devices&rid=$1" | egrep "Temp|Humid" | awk '{print $3 }' | cut -f1 -d\. | grep -v \" | tr -d "\n\r" | sed s/,/%\ /g | awk '{ print $2"° "$1 }'
new.sh script gets the age of the reading from
domoticz1 or domoticz1
Usage: ./new.sh <domoticz-last-numer-ip> <idx> <maxageinseconds>
#!/bin/bash ## server idx time now=$(date +%s) lastupdate=$(curl -s -i -H "Accept: application/json" "http://192.168.1.$1:8080/json.htm?type=devices&rid=$2" | grep LastUpdate | cut -f4 -d\" ) #echo $lastupdate seen=$(date -d "$lastupdate" +%s) #echo $seen #echo "$(( $now - $seen))" difftime="$(( $now - $seen))" if [ $difftime -gt $3 ] ; then echo "WARN : too old - $difftime seconds" exit 1 else echo "OK : $difftime seconds" exit 0 fi
colors2 – a list of colors representing the temperature
red -> green -> blue
255,0,0 255,10,0 255,20,0 255,30,0 255,40,0 255,60,0 255,70,0 255,80,0 255,90,0 255,100,0 255,120,0 255,130,0 255,140,0 255,150,0 255,160,0 255,180,0 255,190,0 255,200,0 255,210,0 255,220,0 255,240,0 255,250,0 253,255,0 215,255,0 176,255,0 101,255,0 62,255,0 23,255,0 0,255,16 0,255,54 0,255,131 0,255,168 0,255,208 0,255,244 0,228,255 0,196,255 0,180,255 0,164,255 0,148,255 0,132,255 0,100,255 0,84,255 0,68,255 0,50,255 0,34,255 0,2,255 0,0,255 1,0,255 2,0,255 3,0,255 5,0,255
Crontab and gif/mp4 generators
# crontab 5 * * * * root /scripts/domotemp/crontemp.sh # crontemp.sh # stores an image with a date. cd /www/webdir/domotemp wget https://mydomoticzweb/domotemp/ -O $(date +%Y%m%d%H).png >/dev/null 2>/dev/null 1>/dev/null # rest scripts mkdir -p embed # below adds the time to the image ls 2020*png | sort -n -k1 | while read ; do hour=$(echo $REPLY | cut -c9,10) ; convert -pointsize 80 -fill black -draw 'text 1650 100 '\"$hour:00\"'' -resize 960x540 $REPLY embed/$REPLY ;done # convert to gif convert $(ls embed/2020*png | sort -n -k1) animation.gif # convert to mp4 ffmpeg -f image2 -r 24 -pattern_type glob -i '*.png' -vcodec libx264 -profile:v high444 -refs 16 -crf 0 -preset ultrafast -vf scale=1920:1080 domotemp.mp4