https://github.com/FreddyVRetro/ISA-PicoMEM
Awesome device
- Ram extender
- Soon Rom emulation
- Floppy and HDD emulation using images on microsd
- NE2000 network card over Wifi!!
- USB Mouse and Joystick
- Adlib
Some other screen captures
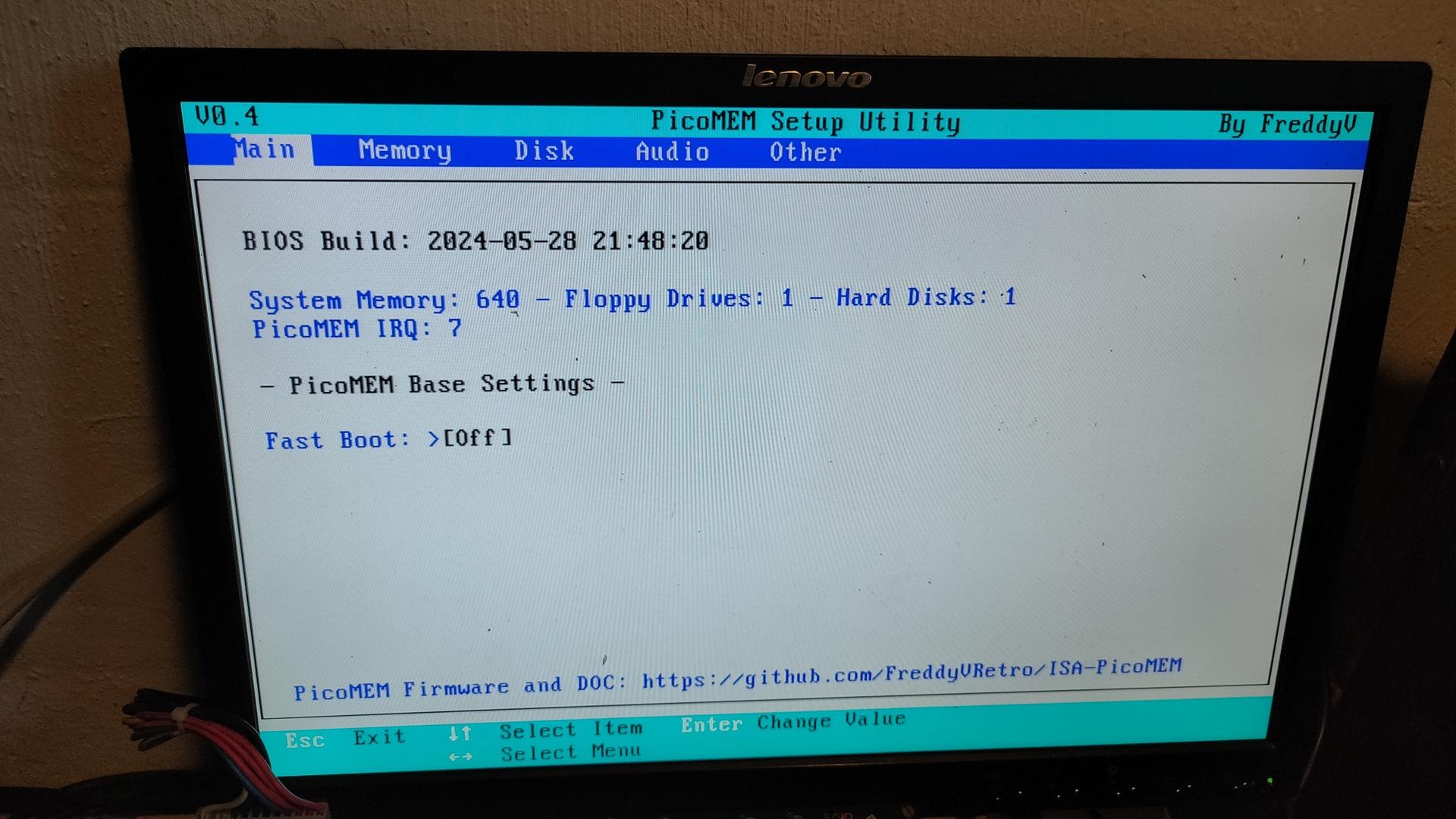
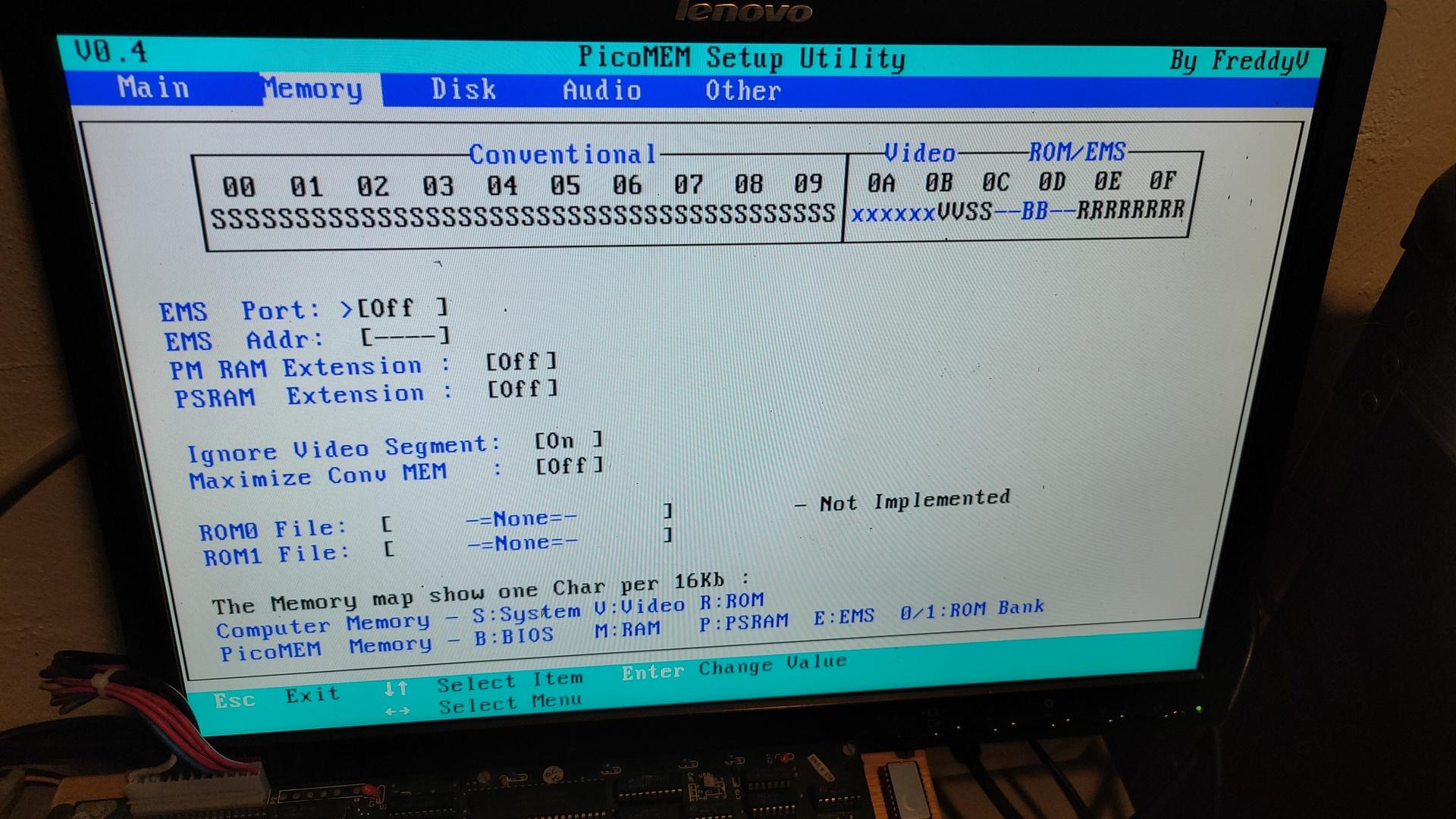
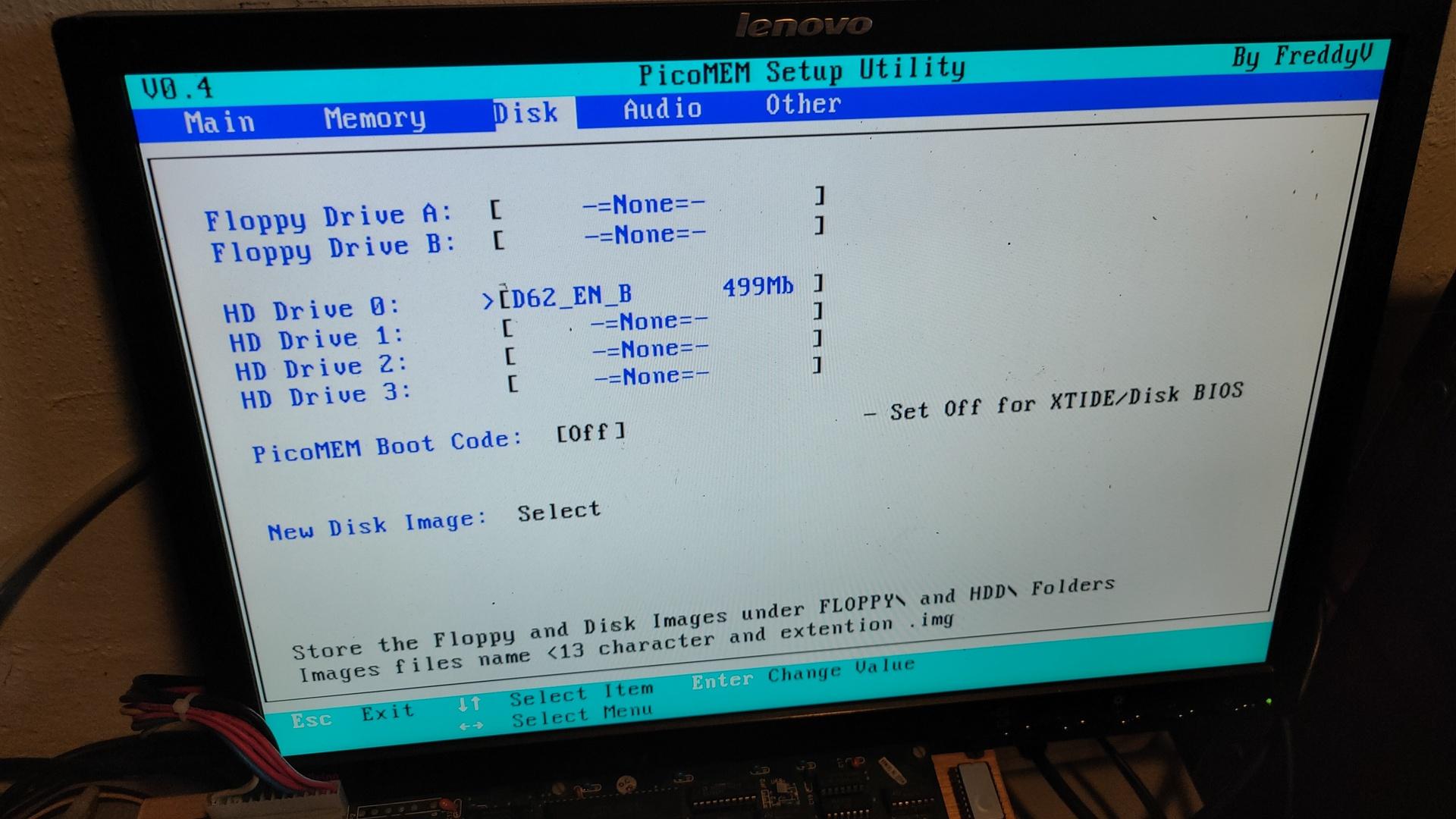
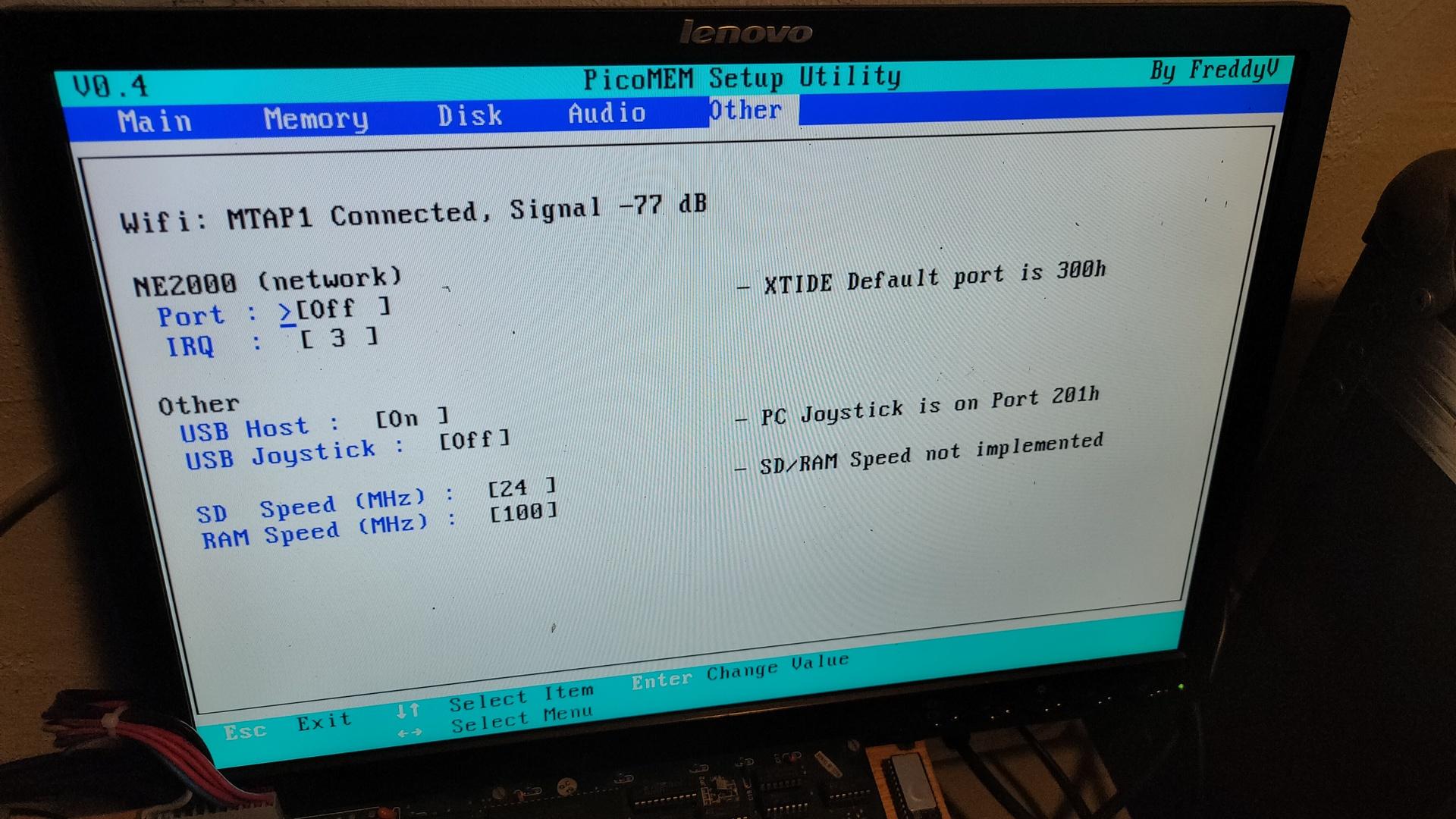
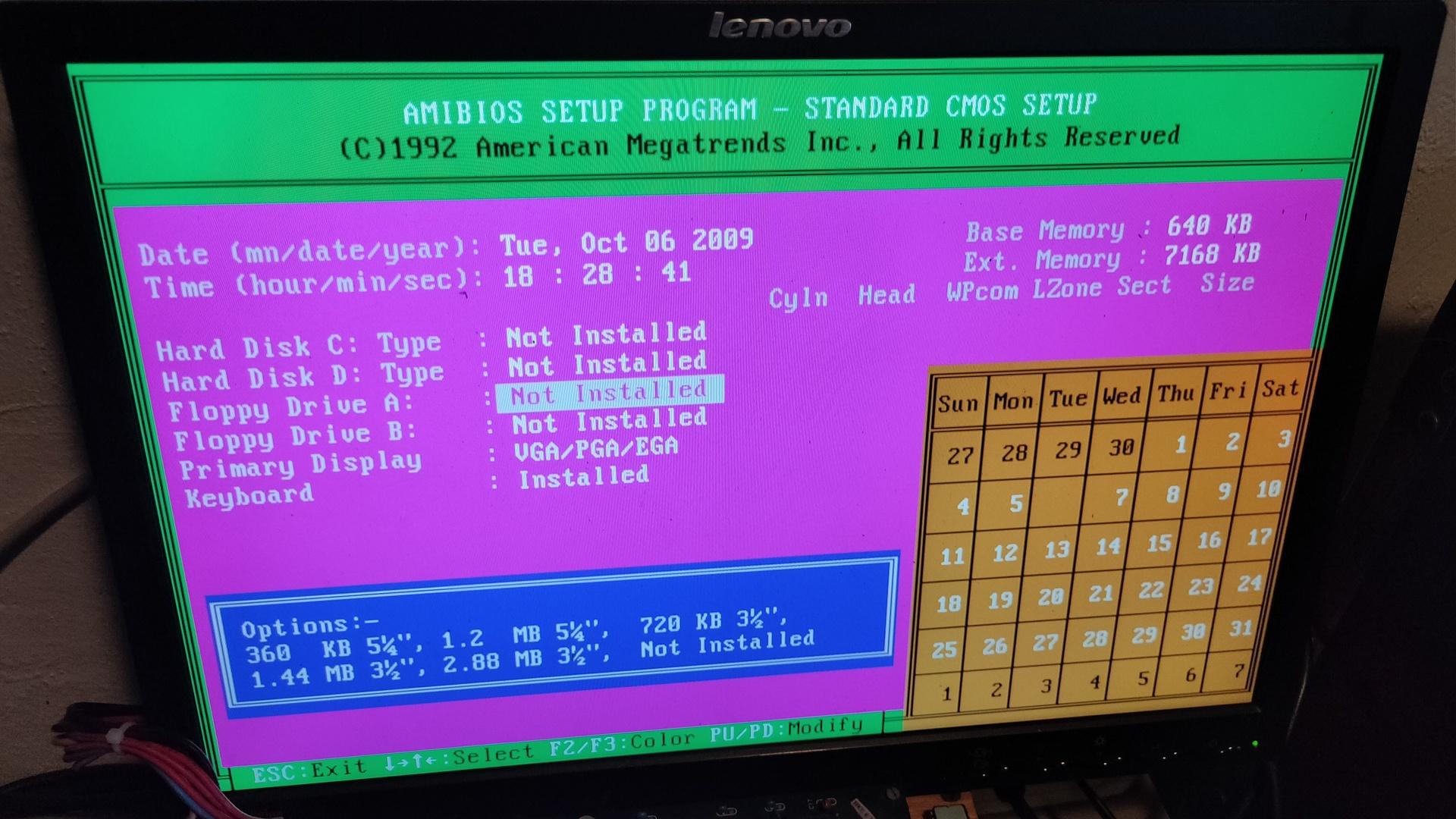
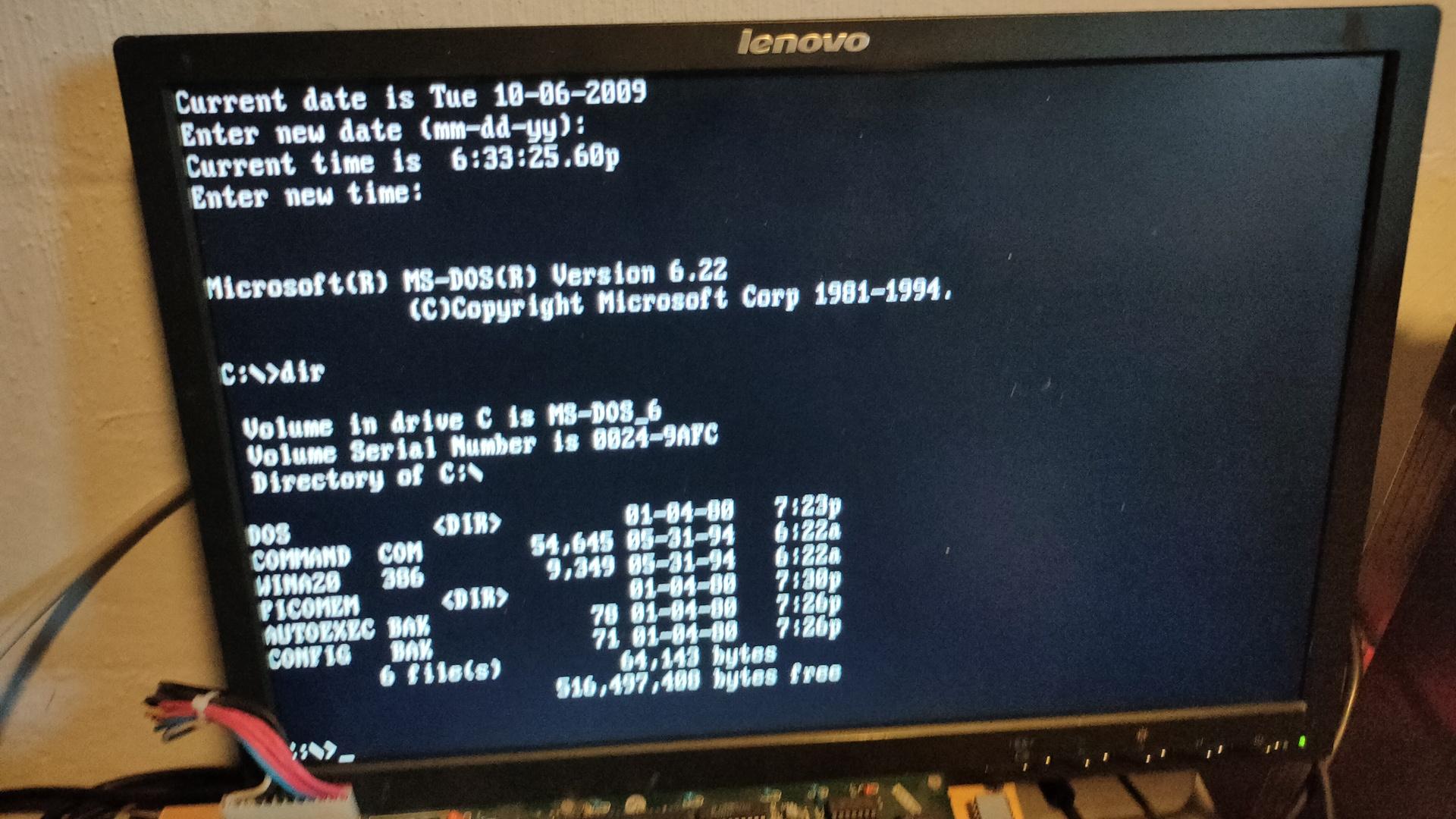
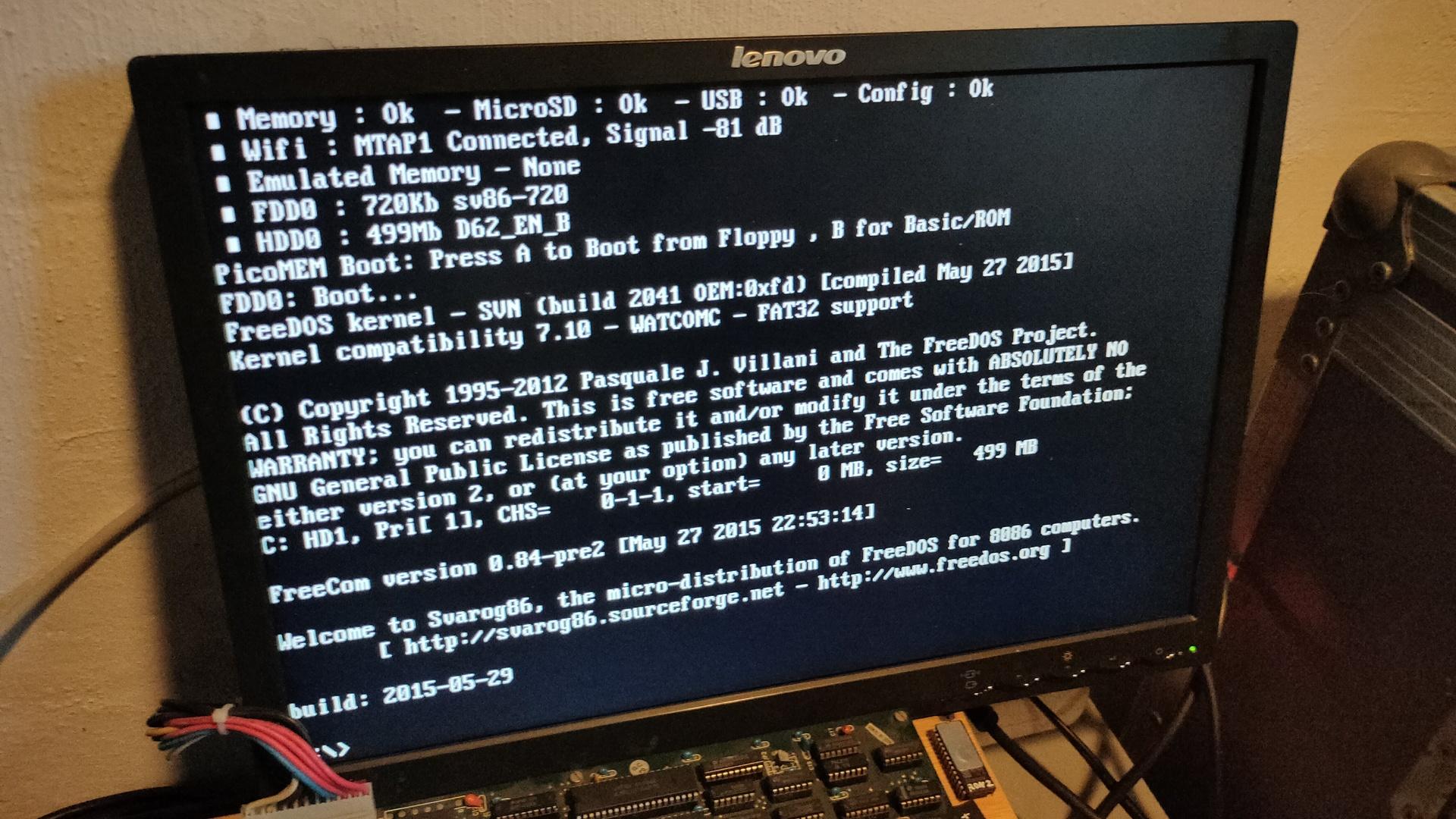
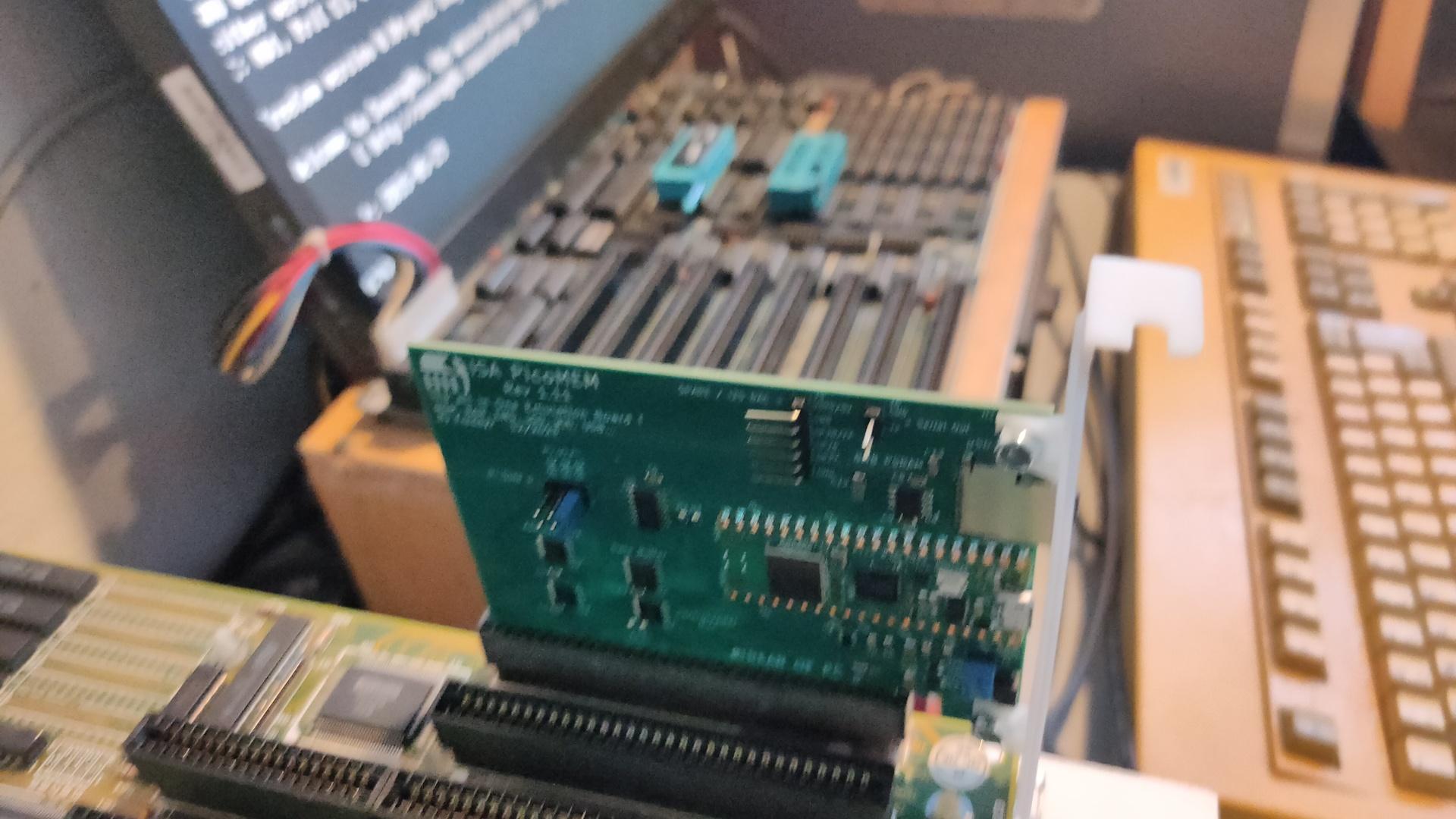
https://github.com/FreddyVRetro/ISA-PicoMEM
Awesome device
Some other screen captures
I’ve been busy programming Python and NodeRed for a client.
But these are the things I’ve done in the last days.
C64 Assembly:
Breaking borders, using sprites and multicolor font intro.
It does not look impressive, but I’ve learned a lot.
Found a new way (for me) to open borders and change border colours on predefined raster lines.
Sources will be posted.
KiCad tutorial, posted on YT also because I could not find many resources about the subject online. Maybe it’s helpful
Video editing using Kdenlive.
Edit: Even faster, use Netlabels, no need to join pins.
Press L (uppercase) select pin 1, name 1.
Press and hold insert until all pins named.
Copy paste socket 5 times and goto your PCB tab.
This movie is about creating a backplane for a 6502 SBC I’m building.
It is real-time and below 4 minutes.
Multi Keyboard
My small multitouch screen came in.
This is for my previously mentioned multi-computer case.
It is going to show multiple keyboard layouts for different systems.
(See previous posts about this)
Waveshare display, Raspberry Zero as HID device, using USB and pin emulated keyboards. (c64 matrix, AT (DIN) keyboard, ps2 keyboard)
Some example screens
I’m also going to make a layout like the keyboards on my 8085
Working on 68000 Single Board Computer.
Made a clock circuit and busy designing a power-on-reset schematic. I’ve made one before, but this circuit needs RESET and HALT being pulled low.
The 68000 being 24 bit address and 16 bit data needs 2x 8-bit roms and 2x 8 bit ram, but i didn’t have the components yet in this picture.
Address decoder using ATF22V10C is also halfway.
Schematics online soon.
Started a protected Git repo for C64 demo and proof of concepts for our old ICECREW group.
Installed Gitea, behind a reverse proxy.
Part of reverse proxy
ProxyRequests Off ProxyPreserveHost On SSLProxyVerify none SSLProxyCheckPeerCN off SSLProxyCheckPeerName off <Location /> ProxyPass http://10.x.y.z:3000/ ProxyPassReverse http://10.x.y.z:3000/ Require ip 213.10.144.27 Require ip a.b.c.d Require ip e.f.g.h </Location>
Gitea config with token login over https
Generate token Login https://icecrew.henriaanstoot.nl/ Select your profile (upper right) And select Settings > Applications Select a name for your token. And press generate Top screen shows a token, copy this! Create new project Press explore (upper left) Select organisation and icecrew Press New Repository, give a name and create (press https when not defaulted, there is NO ssh to this server) The example is wrong! (Use below changing TOKENHERE and PROJECTNAME touch README.md git init -b master git add README.md git commit -m "first commit" git remote add origin https://TOKENHERE@icecrew.henriaanstoot.nl/icecrew/PROJECTNAME.git git push -u origin master Clone a project Goto a project press HTTPS when not defaulted to this. git clone https://icecrew.henriaanstoot.nl/icecrew/borderflag.git edit .git/config and add your token to the url ! to push
My Sidplayer as an option to select own collection.
And I’ve made a top list
# Best composers (no order) Ouwehand_Reyn Tel_Jeroen Huelsbeck_Chris Rowlands_Steve Hubbard_Rob Daglish_Ben Follin_Tim Gray_Matt Tjelta_Geir Mibri (from get in the Van) # Best tunes (no order) R-Type.sid Arkanoid.sid Bottom.sid Turbo_Outrun.sid A_Tune_for_Unity.sid Ohne_Dich_Rammstein.sid # Start of own collection (not in above collection) Abyssus_Ignis_[8580].sid Catastrophe_[8580].sid Dumb_Terminal_[8580].sid Get_in_the_Van_[8580].sid Getting_in_the_Van_[8580].sid Supercharger_[8580].sid Tuna_Guitar_[8580].sid
Investigating syncing effect to Sid music.
I got a great tip from Youth who made the Freakandel demo presented at X2024.
> Setup the loop to play the music > Copy part of the memory to the screen ($0400) in the same loop to look for memory locations that are used as variables for the music. > Looking at > Memory where the music is stored > Zeropage ($00-$ff) > See if there's some useful changes that coincide with for example drums > For my own tunes, I use a music routine where I can put event markers in the music itself and react to those from the code. That's >how I synced https://www.micheldebree.nl/posts/big_angry_sprite/ > You could also try reading the SID registers for voice 3 (waveform and ADSR), those are the only ones that are not write-only. > Obviously you can then only react to those changes in voice 3.
I used retrodebugger to see which bytes are changing.
Then I wrote a program which changes the background colour to this value.
I also made a program to use a joystick to see which address have the most interesting effect.
(use up)
1 !to "sidbgnd.prg",cbm 2 3 * = $0801 4 5 sysline: 6 0801 0b0801009e323036... !byte $0b,$08,$01,$00,$9e,$32,$30,$36,$31,$00,$00,$00 ;= SYS 2061 7 8 * = $080d 9 10 080d 78 sei 11 080e a960 lda #<irq 12 0810 a208 ldx #>irq 13 0812 8d1403 sta $314 14 0815 8e1503 stx $315 15 0818 a91b lda #$1b 16 081a a200 ldx #$00 17 081c a07f ldy #$7f 18 081e 8d11d0 sta $d011 19 0821 8e12d0 stx $d012 20 0824 8c0ddc sty $dc0d 21 0827 a901 lda #$01 22 0829 8d1ad0 sta $d01a 23 082c 8d19d0 sta $d019 24 082f a900 lda #$00 25 0831 200010 jsr $1000 26 0834 58 cli 27 0835 a920 lda #$20 28 0837 8d6b08 sta vector 29 083a a917 lda #$17 30 083c 8d6c08 sta vector+1 31 083f a000 ldy #$00 32 0841 b93017 hold lda $1730,y 33 0844 8d20d0 sta $D020 34 0847 ad00dc lda $dc00 35 084a 2901 and #$1 36 084c c901 cmp #$1 37 084e f0f1 beq hold 38 0850 ad00dc lda $dc00 39 0853 2901 and #$1 40 0855 c900 cmp #$0 41 0857 f0e8 beq hold 42 0859 c8 iny 43 085a 8c6d08 sty vector+2 44 085d 4c4108 jmp hold 45 irq 46 0860 a901 lda #$01 47 0862 8d19d0 sta $d019 48 0865 200310 jsr $1003 49 0868 4c31ea jmp $ea31 50 51 vector 52 086b 0000 !byte $00,$00 53 54 * = $1000 55 1000 4c37104c85104c2f... !binary "Techno_Drums.sid" ,, $7c+2
A fun experiment using opening the C64 border and changing colors on certain rasterlines.
Code (acme)
acme borderflag.asm
x64 +drive8truedrive borderflag.prg
!cpu 650rasterline !to "borderflag.prg",cbm * = $0801 !byte $0d,$08,$dc,$07,$9e,$20,$34 !byte $39,$31,$35,$32,$00,$00,$00 * = $c000 sei ; turn off interrupts ldx #1 ; enable raster interrupts stx $d01a lda #<irq ; set raster interrupt vector ldx #>irq sta $0314 stx $0315 ldy #$f0 ; set first interrupt rasterline sty $d012 lda $d011 ; reset rasterline hi bit and #%01111111 sta $d011 asl $d019 ; ack VIC interrupts cli loop_until_doomsday jmp loop_until_doomsday irq asl $d019 ; ack irq lda #$01 ; set screenframe and background sta $d020 lda #$02 sta $d021 lda #$38 ; wait for line $38 cmp $d012 bne *-3 lda #$02 ; set screenframe and background sta $d020 lda #$01 sta $d021 lda #$f9 ; wait for line $f9C cmp $d012 ; just below border in 25 row mode bne *-3 lda $d011 ; switch to 24 row mode ($d011 bit 3 = 0) and #$f7 ; %11110111 sta $d011 lda #$fd ; wait for line $fd cmp $d012 ; just below border in 25 row mode bne *-3 lda $d011 ; switch back to 25 row mode ($d011 bit 3 = 1) ora #$08 ; %00001000 sta $d011 jmp $ea31 ; exit irq
Started working on my breadboard version of a 68k computer.
When it’s working, I’ll make a PCB version.
Using almost only parts I still have. (No 8mhz crystal)
The 68000 being 24 bit address and 16 bit data needs 2x 8-bit roms and 2x 8 bit ram, but i didn’t have the components yet in this picture.
While tinkering with above, my Fatter Agnus chip came in.
To make a 1mb chipmem version of your rev 5 amiga (PAL)
You need to have a newer version of the Agnus chip (I had 8371, and bought a 8372a) AND you need a 512kb trapdoor memory expansion.
An unmodded rev 5 will see 512kb Chip mem and 512 Fast mem.
Replacing the Agnus 8371 for 8372a:
I lost my PLCC puller, so I modded a paperclip into a puller 🙂
When placing the new chip, I had to tape pin 41 for PAL version.
I used Polyimide Film tape.
Next I had to cut the jumper 2 connection and solder the other pads.
(Bottom and middle disconnect and middle and top bridged)
Next was another cut on the PCB, this disables the trapdoor card detection.
Success!
I think we’ve seen Maderia .. 🙂
I bought a little notebook while being there.
I wrote about 12 pages of ideas, schematics and projects to start.
8085 Cartridge revisited
Problem with cartridge: prg is 17k, exomized 10k.
So you need 2 banks of 8k.
This disables basic rom, needed for the program.
The program needs to be relocated to 0x800 anyway.
So my exomizer options will take care of that.
But the basic is not being enabled again.
exomizer sfx sys -o data.exo -Di_ram_enter=\$37 -Di_ram_during=\$34 -f'LDA #$37 STA $01' 8085.prg xa frame.asm -o frame.bin x64 -cart16 frame.bin
Result … JAM
While I’m not really a gamer, I played a very old game I loved playing on my Amiga.
Its Ports of Call.
Are there others like this game?
Ports of Call is a 1986 business simulation game developed by German duo Rolf-Dieter Klein and Martin Ulrich, and published by Aegis Interactive Entertainment.
The game simulates the management of a global freight transport company, where the player charters freight, and, using the accumulated profit, can buy more and better ships. Minigames include manually piloting your ship into a specified berth in the harbour and picking up survivors from a life-raft.
I loved the manoeuvring part, especially the large ships with both front and back rudders.
Here are some screenshots from Amiga Forever emulator
Post about other old games:
Test picture of a multiprocessor computer setup.
Using buttons on the right, I want the possibility to change between systems and keyboard settings.
Also, multiple software/OS slots for SDCards will be on the right.
The lid containing the keyboard has a handle!
After laser cutting a nice front, it could become a nice road warrior hacking station.
I’m going to replace the wireless keyboard, probably with a touch display and a programmable layout for keyboards.
Something like below
Some layouts:
I’ll probably buy this one from waveshare
Info about Faux86
Re-learning the little I knew (I never had a c64 as a kid).
Back to basics, welll machine code I mean.
Programming a little demo using acme.
Split screen bitmap and text mode plus sid music
Some useful commands
; Dump prg with offset 0x800 per byte and skip 00 00 lines
xxd -o 0x800 -g1 icecrew.prg | uniq -f10
; Write symbol list
acme -l icecrew.sym icecrew.asm
; png to kla (koala picture)
retropixels icecrew.png -o icecrew.kla
; relocate a sid address
sidreloc -r org.sid new.sid
Below code has some flaws:
Many empty gaps, creating a large file.
Exomizer could fix this, but better memory management should be the better solution.
The Koala file has many 0 bytes, the logo is small but the file is created for a full screen image.
!cpu 6502 !to "icecrew1.prg",cbm ; Standard basic sys runner basic_address = $0801 ; sid addresses ; address moved using ; sidreloc -r Lameness_Since_1991.sid lame.sid ; addresses found using ;sidplay2 -v lame.sid ;+------------------------------------------------------+ ;| SIDPLAY - Music Player and C64 SID Chip Emulator | ;| Sidplay V2.0.9, Libsidplay V2.1.1 | ;+------------------------------------------------------+ ;| Title : Lameness Since 1991 | ;| Author : Peter Siekmann (Devilock) | ;| Released : 2017 Oxyron | ;+------------------------------------------------------+ ;| File format : PlaySID one-file format (PSID) | ;| Filename(s) : lame.sid | ;| Condition : No errors | ;| Playlist : 1/1 (tune 1/1[1]) | ;| Song Speed : 50 Hz VBI (PAL) | ;| Song Length : UNKNOWN | ;+------------------------------------------------------+ ;| Addresses : DRIVER = $1C00-$1CFF, INIT = $0FFF | ;| : LOAD = $0FFF-$1B25, PLAY = $1003 | ;| SID Details : Filter = Yes, Model = 8580 | ;| Environment : Real C64 | ;+------------------------------------------------------+ ; sid_address = $0fff sid_play = $1003 sid_init = $0fff ; Character char_address = $3800 screen_mem = $4400 ; Koala address bitmap_address = $6000 bitmap_data = $7f40 bitmap_color = $8328 bitmap_bgcolor = $8710 program_address = $c000 color_mem = $d800 reg_d011 = $D011 ; VIC register ;Bit 7 (weight 128) is the most significant bit of the VIC's nine-bit raster register (see address 53266). ;Bit 6 controls extended color mode ;Bit 5 selects either the text screen ("0") or high resolution graphics ("1"). ;Bit 4 controls whether the screen area is visible or not. ;Bit 3 selects 25 (when set to "1") or 24 (when set to "0") visible character lines on the text screen. ;Bit 0–2 is used for vertical pixel-by-pixel scrolling of the text or high resolution graphics. ; Rom routine to clear screen ( slow ! ) ; Better to do this yourself clear_screen = $e544 * = sid_address !bin "lame.sid",,$7c+2 ; standard charset * = char_address !bin "charset.chr" ; drawn with gimp converted using retropixel ; retropixels icecrew.png -o icecrew.kla * = bitmap_address !bin "icecrew.kla",,$02 ; sys 49152 * = basic_address !byte $0d,$08,$dc,$07,$9e,$20,$34,$39,$31,$35,$32,$00,$00,$00 * = program_address sei ; init lda #$00 tax tay jsr sid_init jsr clear_screen jsr load_bitmap jsr init_text ldy #$7f sty $dc0d sty $dd0d lda $dc0d lda $dd0d lda #$01 sta $d01a lda reg_d011 and #$7f sta reg_d011 ; move interrupt vector to bitmap lda #<interruptbitmap ldx #>interruptbitmap sta $314 ; Low Address part IRQ vector stx $315 ; High Address part IQR vector ldy #$1b sty reg_d011 lda #$7f sta $dc0d lda #$01 sta $d01a ; trigger interrupt at rasterline 0 lda #$00 sta $d012 cli jmp * interruptbitmap inc $d019 ; trigger interrupt at rasterline 128 lda #$80 sta $d012 lda #<interrupttxt ldx #>interrupttxt sta $314 stx $315 jsr bitmap_mode jmp $ea81 interrupttxt ; ack IRQ inc $d019 ; IRQ at line 0 lda #$00 sta $d012 lda #<interruptbitmap ldx #>interruptbitmap sta $314 stx $315 jsr text_mode jsr sid_play jmp $ea81 bitmap_mode ; bitmap graphics multicolor lda #$3b sta reg_d011 lda #$18 sta $d016 ; switch to video bank 2 ($4000-$7FFF) lda $dd00 and #$fc ora #$02 sta $dd00 lda #$18 sta $d018 rts text_mode ; set text mode hires lda #$1b sta reg_d011 lda #$08 sta $d016 ; switch to video bank 1 ($0000-$3FFF) lda $dd00 and #$fc ora #$03 sta $dd00 ; set charset location ; 7 * 2048 = $3800, set in bits 1-3 of $d018 lda $d018 ora #$0e sta $d018 rts load_bitmap lda bitmap_bgcolor sta $d020 sta $d021 ldx #$00 copy_bmp ; screen memory lda bitmap_data,x sta screen_mem,x lda bitmap_data+256,x sta screen_mem+256,x lda bitmap_data+512,x sta screen_mem+512,x lda bitmap_data+768,x sta screen_mem+768,x ; color memory lda bitmap_color,x sta color_mem,x lda bitmap_color+256,x sta color_mem+256,x lda bitmap_color+512,x sta color_mem+512,x lda bitmap_color+768,x sta color_mem+768,x inx bne copy_bmp rts init_text ldx #$00 copy_txt lda text1,x sta $0400+520,x lda text2,x sta $0400+640,x lda text3,x sta $0400+640+120,x lda #$06 sta color_mem+520,x lda #$0e sta color_mem+640,x lda #$0e sta color_mem+640+120,x inx cpx #$28 bne copy_txt rts text1 !scr " back to oldskool demos in 2024 " text2 !scr " greetings to bigred & tyrone & edk " text3 !scr " a lot to relearn - keep coding! "
Today I tested part loading for a demo.
I wanted this to be a multipart loader, instead of a trackloader.
A trackloader can load sector parts which I would like more.
But the C64Pico can’t do disk images. (Mcume)
2nd reason: While I’ve written a track loader for 8086, I never did it for C64. As a kid I didn’t have a C64, so all knowledge I have is from later years.
I’ve written only a few C64 machinecode programs.
You see the first text from the 1st assemby code, then it will load the second at $2000 and does a jmp to this address.
Second text will but displayed.
While i’ve been using KickAss in the past and some other 6502 compilers, I manly use acme.
Makefile I created to compile, create a C64 diskimage and run the program is as below. (No exomizer tools in this Makefile)
all: acme disk run acme: acme testloader.asm acme 2ndpart.asm disk: c1541 -format diskname,id d64 my_diskimage.d64 -attach my_diskimage.d64 -write loader.prg loader.prg -write 2nd 2nd run: x64 my_diskimage.d64