Last Updated or created 2023-01-26
For a new project i’m using parts of my Photo Manager.
UPDATE: Random photo push from directory in one script.
I will post the Minimal code for the following:
- Caching thumbnail generation
- Drag and drop image for displaying on a kodi instance
- Button to start slideshow of a directory
- Open image in browser with obfuscated image url (not shown in movie)
- Stop image playing
Json RPC used:
# Playing a single file curl -H "content-type:application/json" -i -X POST -d '{"jsonrpc":"2.0","id":1,"method":"Player.Open","params":{"item":{"file":"'.$dir2.'"}}}' http://KODI-IP:8080/jsonrpc &'; # Playing a directory curl -H "content-type:application/json" -i -X POST -d '{"jsonrpc":"2.0","id":1,"method":"Player.Open","params":{"item":{"directory":"/path/'.$dir.'"}}}' http://KODI-IP:8080/jsonrpc &'; # Stop playing (i'm stopping player 1 and 2) You can query which player is active, this works also curl -H "content-type:application/json" -i -X POST -d '{"jsonrpc": "2.0", "method": "Player.Stop", "params": { "playerid": 1 }, "id": 1}' http://10.1.0.73:8080/jsonrpc';
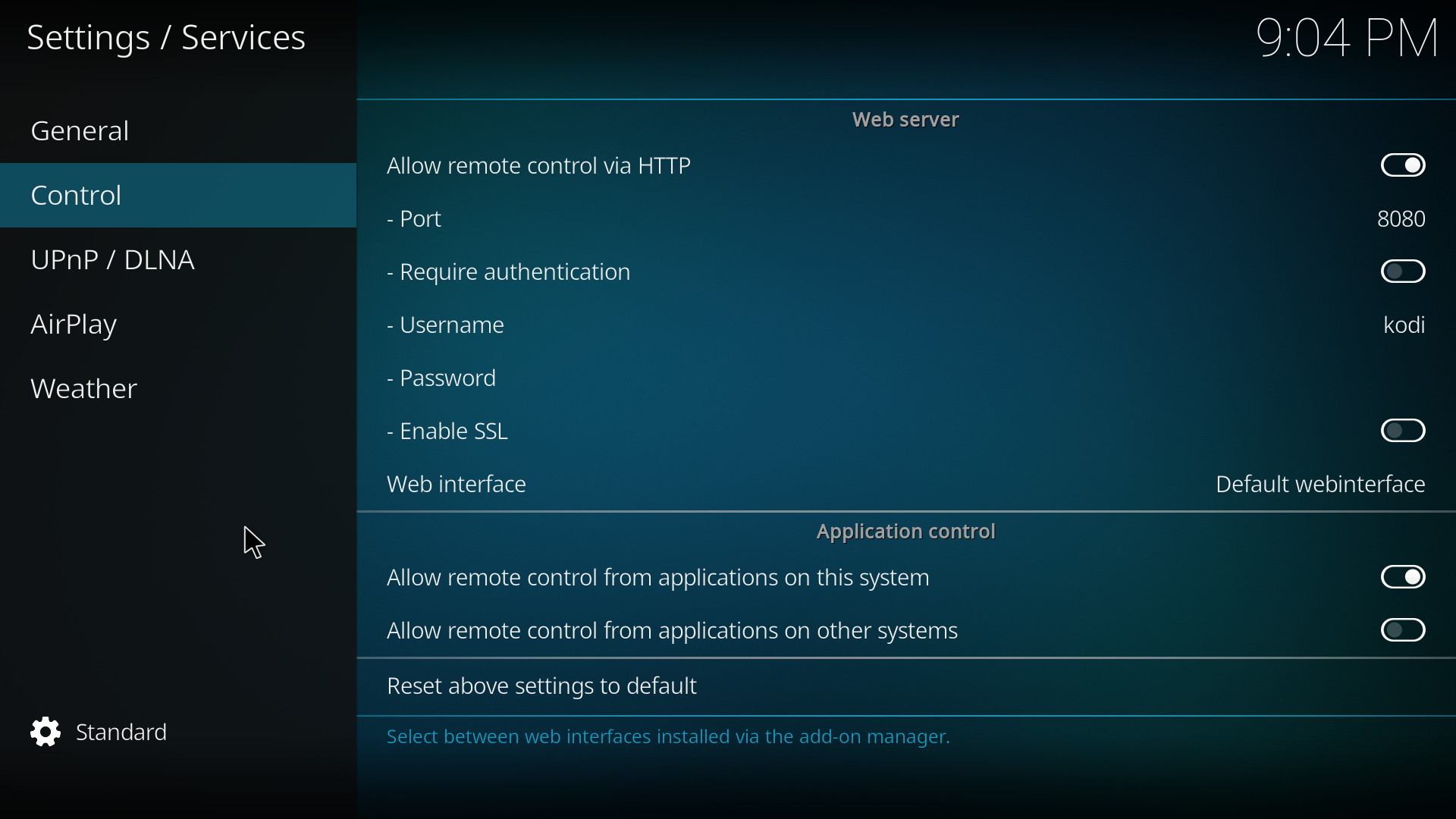
Below the multiple PHP files, i’ve removed a lot of code specific for my manager.
By the way, I love this trick:
header("HTTP/1.1 204 NO CONTENT");
I’ve you put this in the top of your php script which is linked from the first page, you won’t open this link in your browser, but it gets executed nevertheless!
:::::::::::::: push.php :::::::::::::: <!DOCTYPE html> <html> <head> </head> <body> <div id="dropbox">Drop Image</div><br> <a href="playpush.php?dir=TEMP/Sake">playdir</a> <a href="stoppush.php">stop</a> # Here i have a generated part to list my photoalbum photos in this format <a href='getimg.php?imagepath=/mnt/fileserver/TEMP/1.jpg'><img src='getimg2.php?imagepath=/mnt/fileserver/TEMP/1.jpg' width=300 title='1.jpg'></a> <a href='getimg.php?imagepath=/mnt/fileserver/TEMP/2.jpg'><img src='getimg2.php?imagepath=/mnt/fileserver/TEMP/2.jpg' width=300 title='2.jpg'></a> <script type="text/javascript" src="javascript2.js" ></script> </body></html> :::::::::::::: getimg.php - Displays photo in browser (forgotten in movie) :::::::::::::: <?php Header("Content-Type: image/jpeg"); $file = $_GET['imagepath']; $file = str_replace("%20", "\ ", $file); $file = str_replace("(", "\(", $file); $file = str_replace(")", "\)", $file); $log = 'imggetlog'; file_put_contents($log, $file, FILE_APPEND); file_put_contents($log, "\n\r", FILE_APPEND); header('Content-Length: ' . filesize($file)); readfile($file); ?> :::::::::::::: getimg2.php - makes a caching thumbnail /long/image/path/to/photo.jpg -> cachedir/longimagepathtophoto.jpg :::::::::::::: <?php Header("Content-Type: image/jpeg"); $file = $_GET['imagepath']; $file = str_replace("%28", "\(", $file); $file = str_replace("%29", "\)", $file); $file = str_replace("%20", "\ ", $file); $cachename = str_replace("/", "", $file); $cachename = str_replace(" ", "", $cachename); $cachename = "cachedir/$cachename"; $log = 'imggetlog'; file_put_contents($log, $file, FILE_APPEND); file_put_contents($log, "\n\r", FILE_APPEND); if (!file_exists("$cachename")) { exec("convert -resize 300x300 \"$file\" \"$cachename\""); } header('Content-Length: ' . filesize("$cachename")); readfile("$cachename"); ?> :::::::::::::: playpush.php - Pushes DIRECTORY play to Kodi :::::::::::::: <?PHP header("HTTP/1.1 204 NO CONTENT"); header("Cache-Control: no-cache, no-store, must-revalidate"); // HTTP 1.1. header("Pragma: no-cache"); // HTTP 1.0. header("Expires: 0"); // Proxies. $dir=$_GET['dir']; $command='nohup curl -H "content-type:application/json" -i -X POST -d \'{"jsonrpc":"2.0","id":1,"method":"Player.Open","params":{"item":{"directory":"/mnt/fileserver/'.$dir.'"}}}\' http://IPKODI:8080/jso nrpc &'; exec($command, $output, $retval); ?> :::::::::::::: stoppush.php - stops displaying :::::::::::::: <?PHP header("HTTP/1.1 204 NO CONTENT"); header("Cache-Control: no-cache, no-store, must-revalidate"); // HTTP 1.1. header("Pragma: no-cache"); // HTTP 1.0. header("Expires: 0"); // Proxies. $command='curl -H "content-type:application/json" -i -X POST -d \'{"jsonrpc": "2.0", "method": "Player.Stop", "params": { "playerid": 1 }, "id": 1}\' http://IPKODI:8080/jsonrpc'; exec($command, $output, $retval); $command='curl -H "content-type:application/json" -i -X POST -d \'{"jsonrpc": "2.0", "method": "Player.Stop", "params": { "playerid": 2 }, "id": 1}\' http://IPKODI:8080/jsonrpc'; exec($command, $output, $retval); ?> :::::::::::::: javascript2.js :::::::::::::: function getAllElementsWith(tag, attribute, value) { var matchingElements = []; var allElements = document.getElementsByTagName(tag); for (var i = 0; i < allElements.length; i++) { if (value.indexOf(allElements[i].getAttribute(attribute)) != -1) { // Element exists with attribute. Add to array. matchingElements.push(allElements[i]); } } return matchingElements; } // onDrop function onDrop(evt) { evt.stopPropagation(); evt.preventDefault(); var imageUrl = evt.dataTransfer.getData("URL"); var links = getAllElementsWith("a", "href", imageUrl); var image; console.log(links, evt); if(links.length){ image = links[0].getElementsByTagName("img"); if(image.length) imageUrl = image[0].getAttribute("src"); else imageUrl = "#no-image"; } /// alert(imageUrl); var res = imageUrl.replace(/getimg/, "pushplay2"); location.href = (res); }; // onDragOver function onDragOver(evt){ evt.preventDefault(); } var dropbox = document.getElementById('dropbox'); dropbox.addEventListener('drop', onDrop); dropbox.addEventListener("dragover", onDragOver, false);
Random picture push
file=$(find /mnt/fileserver/examples -type f | shuf | head -1) post_data="{\"jsonrpc\":\"2.0\",\"id\":1,\"method\":\"Player.Open\",\"params\":{\"item\":{\"file\":\"$file\"}}}" curl --user user:pass -H "content-type:application/json" -i -X POST --data "${post_data}" http://KODI-IP:8080/jsonrpc
Below a example of what your can do with the code above