Last Updated or created 2024-08-04
Last week I bought an old Bornhack Badge. I thought it needed a display.
Using a SSD1306 display, and Circuitpython I made this.
( Wooded thingy contains an RFID chip ( Part of my player ))
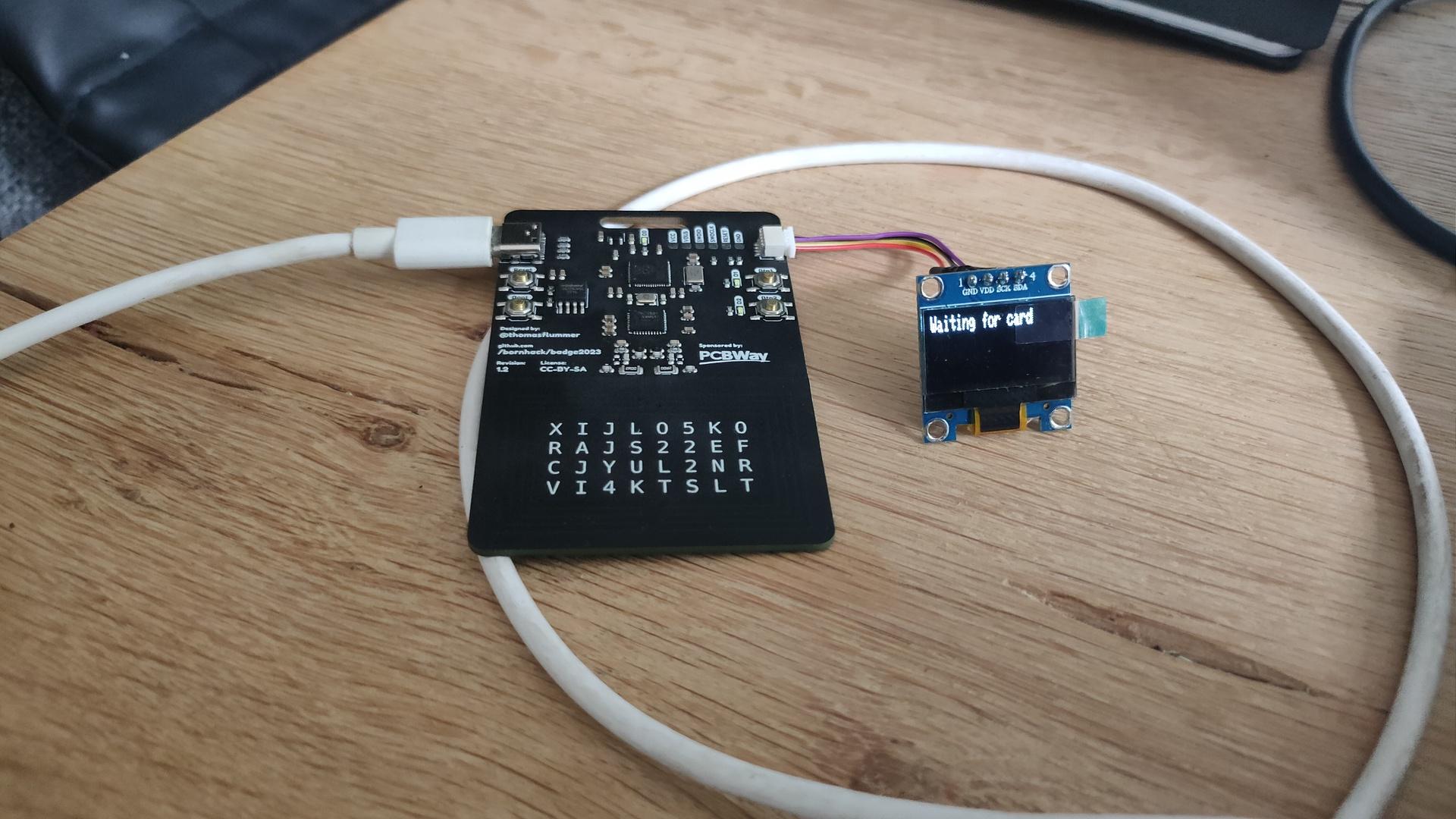
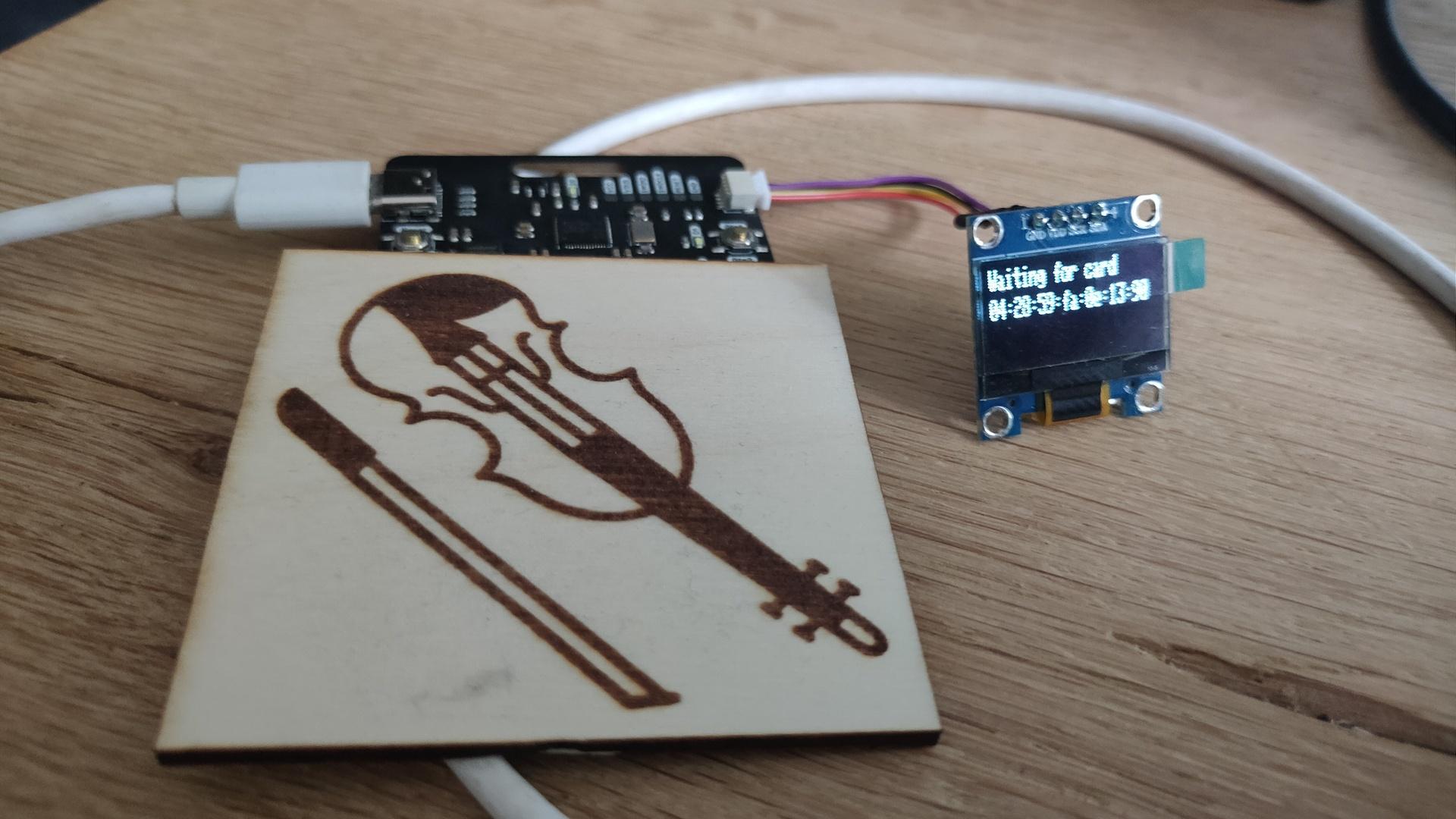
Library and files needed:
- font5x8.bin in root of filesystem ( just google for this file )
- copy of adafruit_framebuf.mpy in /lib
- copy of adafruit_ssd1306.mpy in /lib
Code: (midway some pixel examples, just uncomment)
import board from time import sleep import busio from PN7150 import PN7150 import adafruit_ssd1306 import math import adafruit_framebuf if True: # Fast 400KHz I2C i2c = busio.I2C(board.SCL, board.SDA, frequency = 400000) else: # Regular 100kHz I2C i2c = board.I2C() WIDTH = 32 HEIGHT = 8 buffer = bytearray(round(WIDTH * math.ceil(HEIGHT / 8))) fb = adafruit_framebuf.FrameBuffer( buffer, WIDTH, HEIGHT, buf_format=adafruit_framebuf.MVLSB ) nfc = PN7150(i2c, board.IRQ, board.VEN) display = adafruit_ssd1306.SSD1306_I2C(128, 32, i2c,addr=0x3c) assert nfc.connect() print("Connected.") assert nfc.modeRW() print("Switched to read/write mode.") display.fill(0) display.show() #display.fill(0) #display.text('Hello', 0, 0, 1 ) #display.text('World', 0, 10, 1) #display.show() # Set a pixel in the origin 0,0 position. #display.pixel(0, 0, 1) # Set a pixel in the middle 64, 16 position. #display.pixel(64, 16, 1) # Set a pixel in the opposite 127, 31 position. #display.pixel(127, 31, 1) #display.show() while True: display.fill(0) display.text('Waiting for card', 0, 0, 1 ) display.show() assert nfc.startDiscoveryRW() print("Waiting for card..") card = nfc.waitForCard() assert nfc.stopDiscovery() print("ID: {}".format(card.nfcid1())) id = card.nfcid1() display.text(id, 0, 10, 1 ) display.show() sleep(0.5)
Not sure about display i2c address? Use below code
import time import board import busio # List of potential I2C busses ALL_I2C = ("board.I2C()",) # Determine which busses are valid found_i2c = [] for name in ALL_I2C: try: print("Checking {}...".format(name), end="") bus = eval(name) bus.unlock() found_i2c.append((name, bus)) print("ADDED.") except Exception as e: print("SKIPPED:", e) # Scan valid busses if len(found_i2c): print("-" * 40) print("I2C SCAN") print("-" * 40) while True: for bus_info in found_i2c: name = bus_info[0] bus = bus_info[1] while not bus.try_lock(): pass print( name, "addresses found:", [hex(device_address) for device_address in bus.scan()], ) bus.unlock() time.sleep(2) else: print("No valid I2C bus found.")