Last Updated or created 2025-02-17
My proof of concept to build an electronic chanter for less than 4 euros.
It uses a Lolin32 Lite and a buzzer. (No libraries needed)
(Some wires, thumbtacks and a PVC tube.)
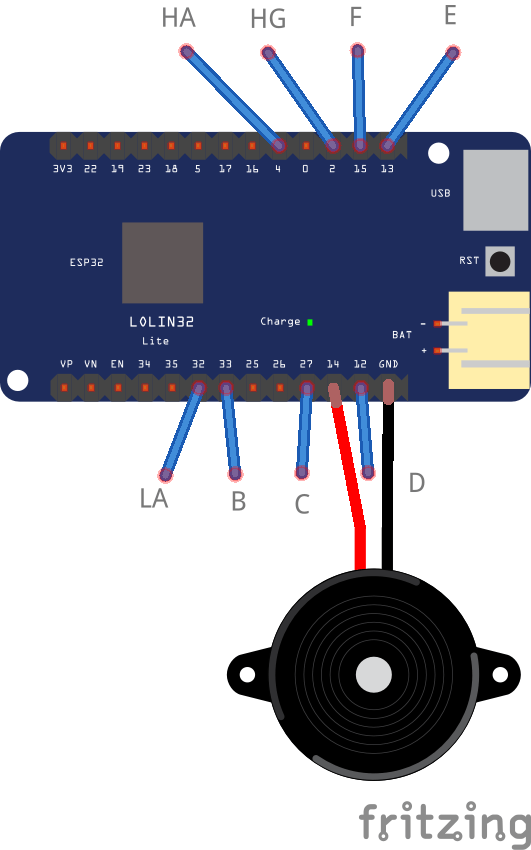
Plays accidentals (false fingering)
Vibrato
Has serial console debugging
Need other frequencies? Just edit the source.
CODE
int prevnote = 0; // Note freq table int ha = 880; int hg = 783; int fs = 739; int f = 698; int e = 659; int d = 587; int cs = 554; int c = 523; int b = 493; int as = 466; int la = 440; int lg = 391; int mute = 0; // 8 Bits to note, luckly not 9 fingers :) // 0 (00000000) = all fingers off = mute // bottom hand top hand // 0111 011 // 1 // 01110111 = 119 = E // 255 (11111111) = all fingers on = lg // below per 10 all 255 posibilities // Look at position 175 // custom frequency to get vibrato on D // using bottom hand middle finger. int data[] = { mute,hg,ha,fs,ha,hg,ha,e,ha,hg,ha, f,ha,hg,ha,d,ha,hg,ha,fs,ha, hg,ha,e,ha,hg,ha,f,ha,hg,ha, c,ha,hg,ha,f,ha,hg,ha,e,ha, hg,ha,f,ha,hg,ha,d,ha,hg,ha, f,ha,hg,ha,e,ha,hg,ha,f,ha, hg,ha,b,ha,hg,ha,f,ha,hg,ha, e,ha,hg,ha,f,ha,hg,ha,d,ha, hg,ha,f,ha,hg,ha,e,ha,hg,ha, e,ha,hg,ha,c,ha,hg,ha,f,ha, hg,ha,e,ha,hg,ha,f,ha,hg,ha, d,ha,hg,ha,fs,ha,hg,ha,e,ha, hg,ha,f,ha,hg,ha,la,ha,hg,ha, f,ha,hg,ha,e,ha,hg,ha,f,ha, hg,ha,d,ha,hg,ha,f,ha,hg,ha, e,ha,hg,ha,f,ha,hg,ha,cs,ha, hg,ha,f,ha,hg,ha,e,ha,hg,ha, f,ha,hg,ha,580,ha,hg,ha,f,ha, hg,ha,e,ha,hg,ha,f,ha,hg,ha, as,ha,hg,ha,f,ha,hg,ha,e,ha, hg,ha,f,ha,hg,ha,d,ha,hg,ha, f,ha,hg,ha,e,ha,hg,ha,f,ha, hg,ha,c,ha,hg,ha,fs,ha,hg,ha, e,ha,hg,ha,f,ha,hg,ha,d,ha, hg,ha,fs,ha,hg,ha,e,ha,hg,ha, fs,ha,hg,ha,lg }; void setup() { pinMode(14, OUTPUT); Serial.begin(115200); delay(1000); } void loop() { int t1=touchRead(4); int t2=touchRead(2); int t3=touchRead(15); int t4=touchRead(13); int t5=touchRead(12); int t6=touchRead(27); int t7=touchRead(33); int t8=touchRead(32); int note = 0; // Debug reading //Serial.println(t1); // My readings are near zero and above 50 // So I chose 30 (adjust when needed) if ( t1 < 30) { bitSet(note, 0); } if ( t2 < 30) { bitSet(note, 1); } if ( t3 < 30) { bitSet(note, 2); } if ( t4 < 30) { bitSet(note, 3); } if ( t5 < 30) { bitSet(note, 4); } if ( t6 < 30) { bitSet(note, 5); } if ( t7 < 30) { bitSet(note, 6); } if ( t8 < 30) { bitSet(note, 7); } //Serial.println(note); if (note == 0 && note != prevnote) { noTone(14); prevnote = 0; } if (note != prevnote) { tone(14,data[note]); // debug //Serial.print("Note number : "); //Serial.println(note); //Serial.print("Freq : "); //Serial.println(data[note]); prevnote = note; } }