I started to get some composite video generated with a arduino for my 6502 project.
UPDATE: 20221021
It is based on Grant Searle’s design, and yesterday I had some signals on my scope which looked like a screen with a character. But my monitor would not recognize a usable signal.
Today I tried a second version and another set of chips and crystals.
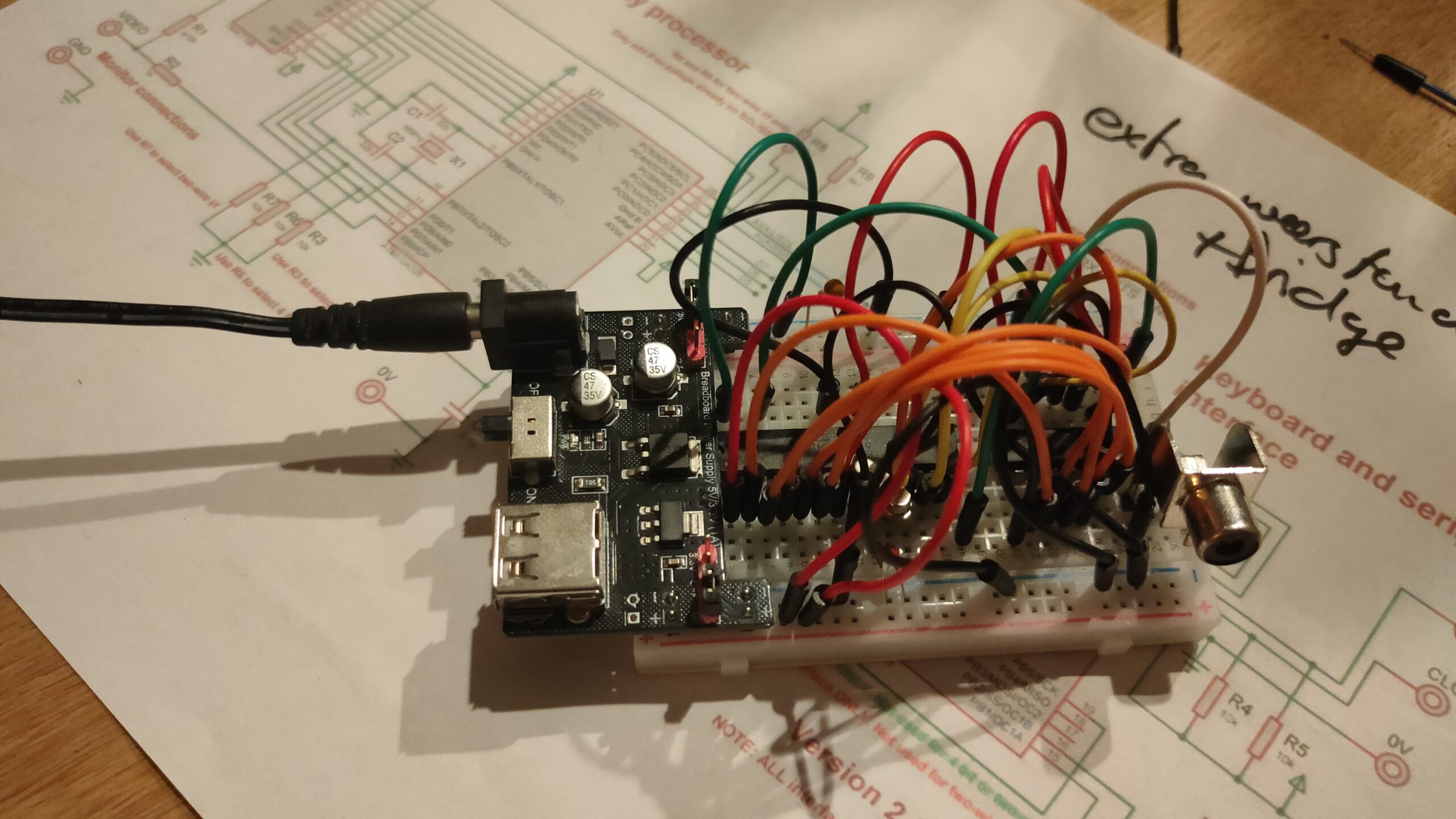
It looks like a signal, but I can’t see a clock pulse from the crystal?! So .. how?
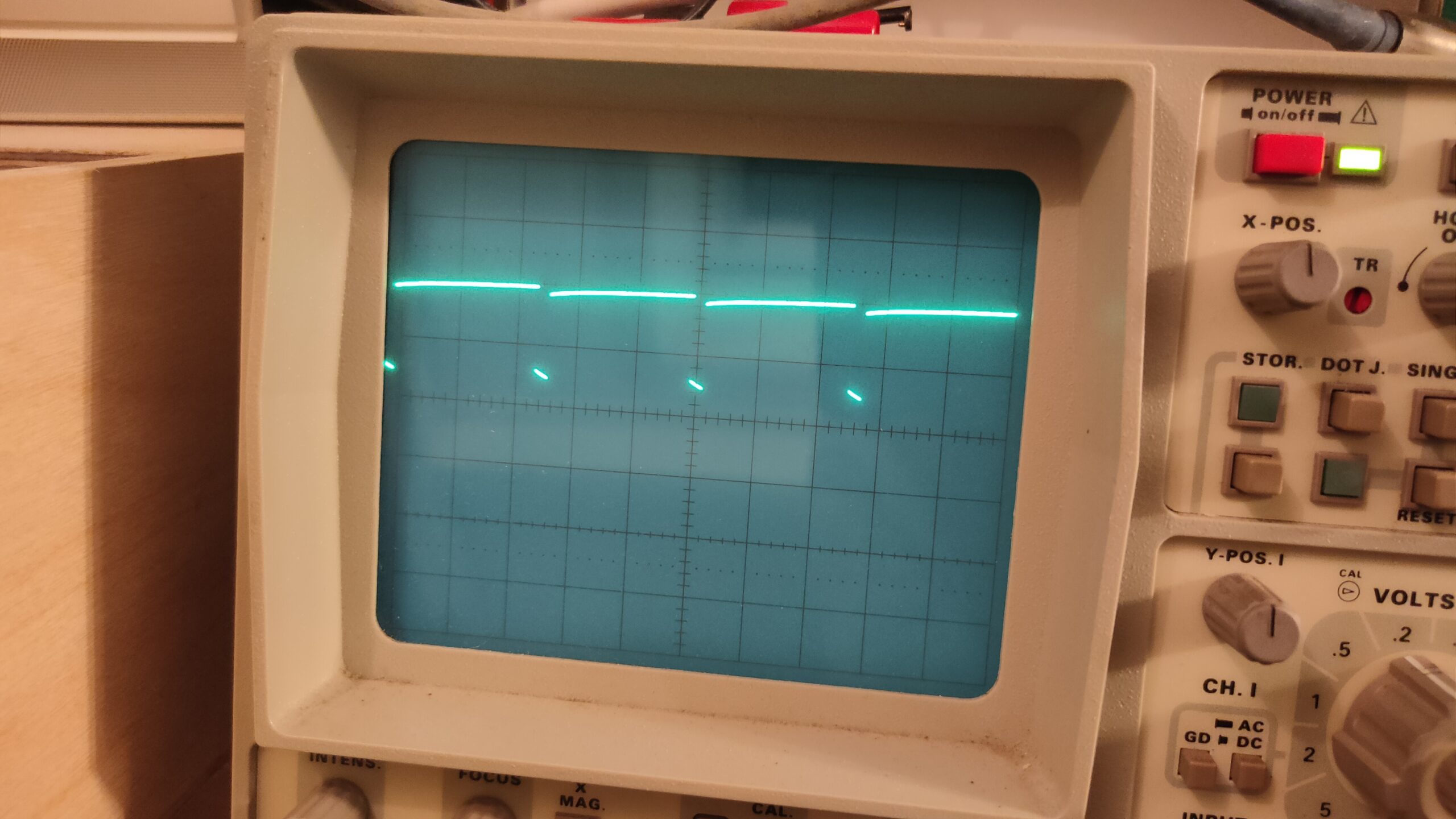
Maybe I used a bad power supply. And killed something?
UPDATE: 20221021
After switching to another power supply, and checking the atmega328p fuses again (also wrong) .. at least SOME success!
Some minipro info #Erase minipro -p ATMEGA328P@DIP28 -E #Flash hex code minipro -p ATMEGA328P@DIP28 -w SBCVideo.hex #Flash fuses minipro -p ATMEGA328P@DIP28 -e -c config -w fuses #Used fuses file lfuse = 0xf7 hfuse = 0xd9 efuse = 0xfc lock = 0xff lfuse = 0xff hfuse = 0xff efuse = 0xff user_id0 = 0xff user_id1 = 0xff user_id2 = 0xff user_id3 = 0xff user_id4 = 0x37 user_id5 = 0x37 user_id6 = 0x39 user_id7 = 0x36 #Dump all from atmega328p minipro -p ATMEGA328P@DIP28 -r dump -f ihex
Some info about the fuses:
https://www.allaboutcircuits.com/projects/atmega328p-fuse-bits-and-an-external-crystal-oscillator