Last Updated or created 2024-06-27
Testing the first keyboard. It is the 8085-SDK hex matrix keyboard.
It is running on a Raspberry Pi Zero 2, without X server.
So the images are displayed using the framebuffer.
Also the touch data is read using evdev and the raw devices.
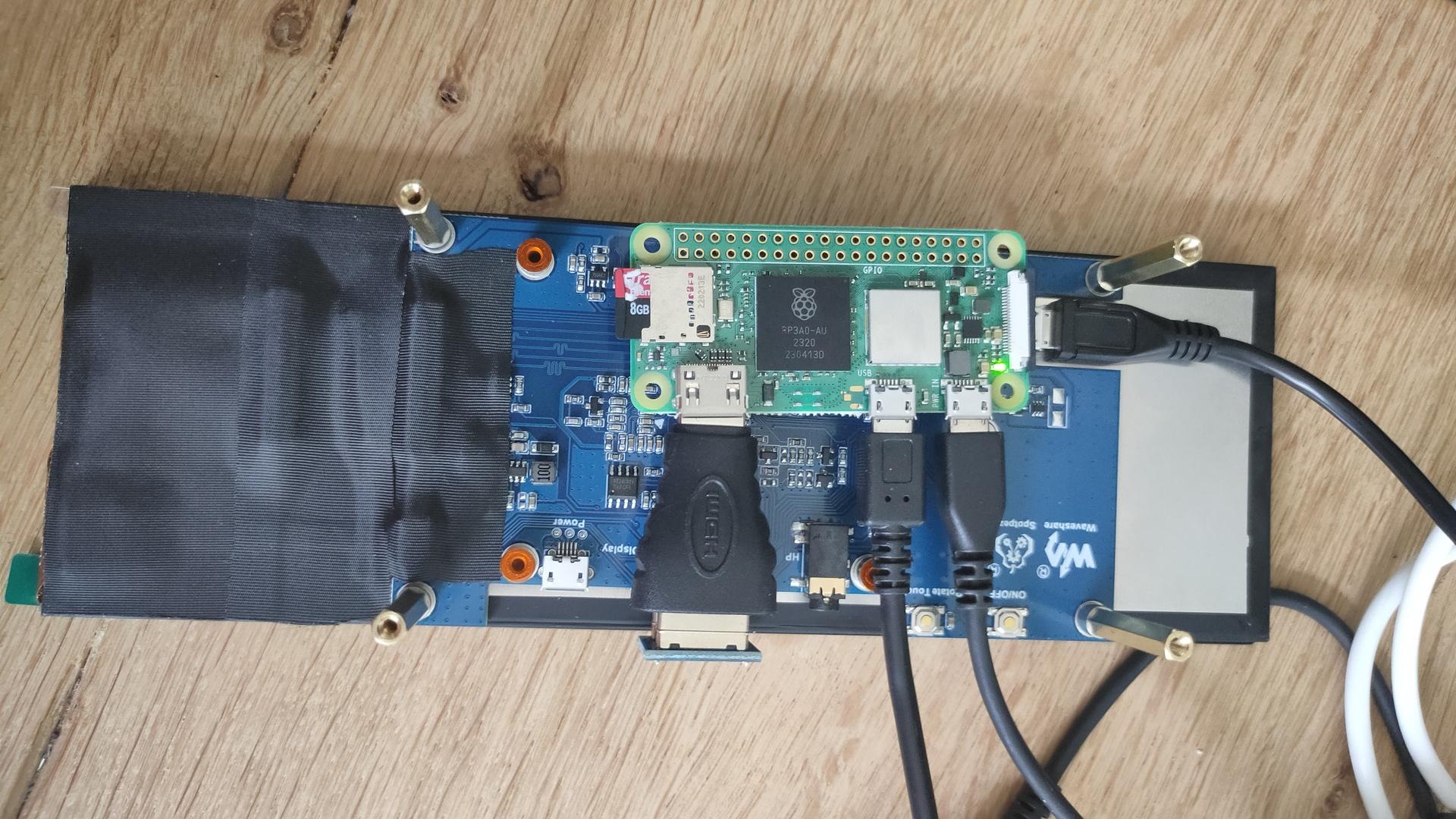
Todo:
- HID part
- Add a rotary button for the selection of the different Keyboard Layouts
- Improvement keyboard matrix calculation to find out which key is being pressed.
- Code to control AT/PS2 computers directly using GPIO pins
- Add a controller to use Raw controlling of matrix pins ( 6502 C64 hardware for example )
Bash test and configuring the OS for testing.
cat <<EOF >> /boot/config.txt hdmi_group=2 hdmi_mode=87 hdmi_timings=400 0 100 10 140 1280 10 20 20 2 0 0 0 60 0 43000000 3 display_rotate=3 EOF # Image testing apt-get install fbi sudo fbi -d /dev/fb0 -T 1 8085.png -a --noverbose apt-get install python3-evdev python3-uinput evtest evtest
First simple python test
import select from math import floor import sys slot = 0 keysname=[["F","E","D","C","vect-int","reset"], ["B","A","9","8","GO","Single-Step"], ["7","6","5","4","Exam-reg","Subst-mem"], ["3","2","1","0","Exec","Next"], ] keysnames=[["F","E","D","C","vect-int","reset"], ["B","A","L","H","GO","Single-Step"], ["PCL","PCH","SPL","SPH","Exam-reg","Subst-mem"], ["3","2","1","0",".",","], ] for path in evdev.list_devices(): device = evdev.InputDevice(path) if evdev.ecodes.EV_ABS in device.capabilities(): break else: sys.stderr.write('Failed to find the touchscreen.\n') sys.exit(1) while True: r, w, x = select.select([device.fd], [], []) id_ = -1 x = y = 0 for event in device.read(): if event.code == event.value == 0: if id_ != -1: yy = floor(( x - 600 ) / 700) xx = floor(( y - 1377 ) / 226) if yy < 4 and yy >=0 and xx < 6 and xx >= 00: if slot == 1: print(keysnames[yy][xx]) else: print(keysname[yy][xx]) elif event.code == ABS_MT_TRACKING_ID: id_ = event.value elif event.code == ABS_MT_SLOT: slot = event.value elif event.code == ABS_MT_POSITION_X: x = event.value elif event.code == ABS_MT_POSITION_Y: y = event.value
I came up with a simple matrix calculation
Pressing the 4 corner keys gave me x and y.
I took averages for min and max reading.
I don’t need pixel-perfect reading, and I noticed values between 960 and 3080 vertically.
We want 960 – 3080 into 4 blocks, but the middle should start @ 960.
So 3080/3 = about 700
700 / 2 = 350
block 1 starts 350 sooner than 960 is ~ 600
Upper key y coords = 600-> + 700
Next is 1300 -> + 700
converting to whole numbers using floor gives me:
floor(( y – 600 ) / 700)
NOTE: My x and y are rotated
Example using coordinates
1600, 1600
floor(( 1600 – 600 ) / 700) = floor(1,4…) = 1st row
(from row 0,1,2,3)