One of the first attempts to do some augmented reality.
Tried to display note buttons realtime on a instrument.
I’m using a image on a screen due to the lighting, i was unable to test my real instrument with the light i had when testing.
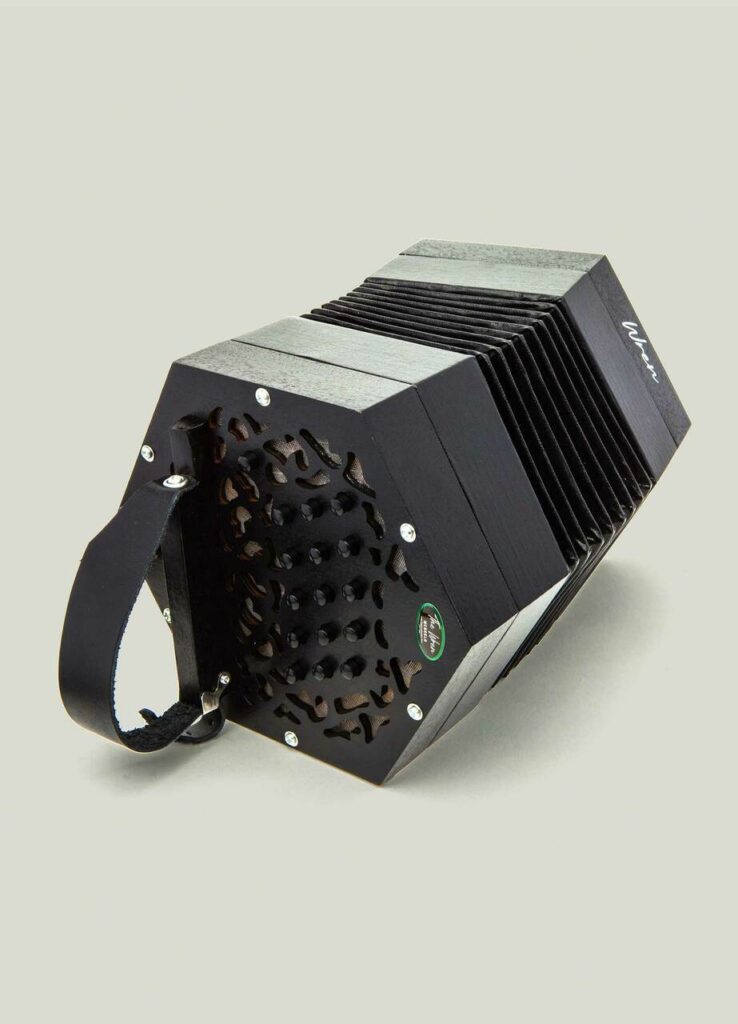
One of the first attempts to do some augmented reality.
Tried to display note buttons realtime on a instrument.
I’m using a image on a screen due to the lighting, i was unable to test my real instrument with the light i had when testing.
I made a little script to make a 360 spherical panorama photo, using a remote controlled IP cam,
Looking at the API CGI, i only needed to control the movement of the camera and getting a snapshot.
After getting all those pictures, i only needed to stitch them using Hugin.
When viewing the image with VR Glasses, i could look around my room, without image distortion, like below flattend example.
The ipcam generates a token, which you have to use in your curl commands.
TOKEN PART
token=$( curl -s -d '[{"cmd":"Login","action":0,"param":{"rs": "abcd", "User":{"userName":"admin","password":"SECRETPASSWORD"}}}]' "http://camera.ip.number.here/cgi-bin/api.cgi?cmd=Login&token=null" -H 'Content-Type: application/json' | grep name | cut -f4 -d\" )
GETTING A IMAGE FROM IPCAM
wget "http://camera.ip.number.here/cgi-bin/api.cgi?cmd=Snap&channel=0&rs=123asd&user=admin&password=SECRETPASSWORD" -O output.jpg
CONTROLLING MOVEMENT
curl -X POST -d '[{"cmd":"PtzCtrl","action":0,"param":{"rs": "abcd", "channel":0,"op":"Left","speed":1,"id":1}}]' "http://camera.ip.number.here/cgi-bin/api.cgi?cmd=PtzCtrl&token=$token"
COMPLETE SCRIPT
#!/bin/bash
token=$( curl -s -d '[{"cmd":"Login","action":0,"param":{"rs": "abcd", "User":{"userName":"admin","password":"SECRETPASSWORD"}}}]' "http://camera.ip.number.here/cgi-bin/api.cgi?cmd=Login&token=null" -H 'Content-Type: application/json' | grep name | cut -f4 -d\" )
echo $token
if [ $2 == "max" ]; then
curl -X POST -d '[{"cmd":"PtzCtrl","action":0,"param":{"rs": "abcd", "channel":0,"op":"'$1'","speed":1,"id":1}}]' "http://camera.ip.number.here/cgi-bin/api.cgi?cmd=PtzCtrl&token=$token"
exit 0
fi
for x in $(seq -w 1 25) ; do
for y in $(seq -w 1 12) ; do
wget "http://camera.ip.number.here/cgi-bin/api.cgi?cmd=Snap&channel=0&rs=123asd&user=admin&password=SECRETPASSWORD" -O ${x}${y}.jpg
curl -X POST -d '[{"cmd":"PtzCtrl","action":0,"param":{"rs": "abcd", "channel":0,"op":"Right","speed":1,"id":1}}]' "http://camera.ip.number.here/cgi-bin/api.cgi?cmd=PtzCtrl&token=$token"
sleep 0.5
curl -X POST -d '[{"cmd":"PtzCtrl","action":0,"param":{"rs": "abcd", "channel":0,"op":"Stop","speed":1,"id":1}}]' "http://camera.ip.number.here/cgi-bin/api.cgi?cmd=PtzCtrl&token=$token"
sleep 5
done
curl -X POST -d '[{"cmd":"PtzCtrl","action":0,"param":{"rs": "abcd", "channel":0,"op":"Left","speed":1,"id":1}}]' "http://camera.ip.number.here/cgi-bin/api.cgi?cmd=PtzCtrl&token=$token"
sleep 30
curl -X POST -d '[{"cmd":"PtzCtrl","action":0,"param":{"rs": "abcd", "channel":0,"op":"Stop","speed":1,"id":1}}]' "http://camera.ip.number.here/cgi-bin/api.cgi?cmd=PtzCtrl&token=$token"
sleep 1
curl -X POST -d '[{"cmd":"PtzCtrl","action":0,"param":{"rs": "abcd", "channel":0,"op":"Up","speed":1,"id":1}}]' "http://camera.ip.number.here/cgi-bin/api.cgi?cmd=PtzCtrl&token=$token"
sleep 0.5
curl -X POST -d '[{"cmd":"PtzCtrl","action":0,"param":{"rs": "abcd", "channel":0,"op":"Stop","speed":1,"id":1}}]' "http://camera.ip.number.here/cgi-bin/api.cgi?cmd=PtzCtrl&token=$token"
sleep 5
done
I’ve got a retro-pi running for some time, now .. posting a little movie.
The gamehat i’ve got a week now.
Retropi met Darish Zone King Image
Own hacks on this system:
I’ve got a bigben controller which i can connect to the Raspberry USB .
We needed to clean up the mess in the serverroom.
GlusterFS (Gluster File System) is an open source Distributed File System that can scale out in building-block fashion to store multiple petabytes of data.
Below is a test environment which creates 5 docker instances, which represent 5 gluster servers.
This was for test repairing our work gluster.
First install gluster and pull a image: docker pull gluster/gluster-centos
gethosts
for f in 1 2 3 4 5;
do
echo "$(docker exec -it gluster_${f} ip a s | grep 172 | awk '{ print $2 }' | cut -f1 -d/) gluster_${f}"
done
create_dockers
for f in 1 2 3 4 5; do
docker run --name gluster_${f} --privileged=true -d gluster/gluster-centos /usr/sbin/init
done
create_bricks
for f in 1 2 3 4 5; do
docker exec -it gluster_${f} mkdir -p /bricks/brick01
done
destroy_dockers
for f in 1 2 3 4 5; do
docker stop gluster_${f}
docker rm gluster_${f}
done
diskcreator
for f in $(seq 1 5); do
dd if=/dev/zero of=/root/disk${f} count=1 bs=100M
losetup /dev/loop${f} /root/disk${f}
docker run --name gluster_${f} --privileged=true --device=/dev/loop${f} -d gluster/gluster-centos /usr/sbin/init
done
lvm-dockers
modprobe dm_thin_pool (in docker)
modprobe dm_thin_pool (ook in VM zelf)
modprobe device-mapper ??
pvcreate /dev/loop0
vgcreate brick01 /dev/loop0
lvcreate -L 50M -T brick01 -n thin_brick01
lvcreate -V 40M -T brick01/thin_brick01 -n testvolume
mkfs -t xfs -i size=512 /dev/brick01/testvolume
mount /dev/brick01/testvolume /bricks/brick01
lvextend -L+10M /bricks/brick01
xfs_growfs /dev/brick01/testvolume
fash@fash-Vortex:~$ cat docker-lvm
modprobe dm_thin_pool (in docker)
modprobe dm_thin_pool (ook in VM zelf)
modprobe device-mapper ??
pvcreate /dev/loop0
vgcreate brick01 /dev/loop0
lvcreate -L 50M -T brick01 -n thin_brick01
lvcreate -V 40M -T brick01/thin_brick01 -n testvolume
mkfs -t xfs -i size=512 /dev/brick01/testvolume
mount /dev/brick01/testvolume /bricks/brick01
lvextend -L+10M /bricks/brick01
xfs_growfs /dev/brick01/testvolume
How to use
./create ./gethosts voor info docker exec -it gluster_1 /bin/bash # GEEN HOSTSNAMES INGEVULD! gluster peer probe 172.17.0.2 gluster peer probe 172.17.0.3 gluster peer probe 172.17.0.4 gluster peer probe 172.17.0.5 Geen persistent storage aangemaakt evt kunnen we ook in de docker zelf testen docker exec -it gluster_1 mkdir -p /bricks/brick01 docker exec -it gluster_2 mkdir -p /bricks/brick01 docker exec -it gluster_3 mkdir -p /bricks/brick01 docker exec -it gluster_4 mkdir -p /bricks/brick01 gluster volume create testvolume 172.17.0.2:/bricks/brick01 172.17.0.3:/bricks/brick01 172.17.0.4:/bricks/brick01 172.17.0.5:/bricks/brick01 force gluster volume start testvolume ### NOG TE TESTEN #gluster volume create testvolume replica 2 172.17.0.2:/bricks/brick01 172.17.0.3:/bricks/brick01 172.17.0.4:/bricks/brick01 172.17.0.5:/bricks/brick01 force ### NOG TE TESTEN #gluster volume create testvolume replica 2 arbiter 1 172.17.0.2:/bricks/brick01 172.17.0.3:/bricks/brick01 172.17.0.4:/bricks/brick01 172.17.0.5:/bricks/brick01 force mount -t glusterfs 172.17.0.2:/testvolume /media/ cd /media touch {1..9} exit for f in 1 2 3 4 ; do echo "gluster_${f}" ; docker exec -it gluster_${f} ls /bricks/brick01 ;done # DESTROY for f in 1 2 3 4 5; do docker stop gluster_${f} docker rm gluster_${f} done
Howto reset-replicated-brick-same-server
Using clean glusterdockers
./create_dockers
./create_bricks
./gethosts
# docker exec -it gluster_1 /bin/bash
# gluster peer probe 172.17.0.2
# gluster peer probe 172.17.0.3
# gluster peer probe 172.17.0.4
# gluster peer probe 172.17.0.5
# Gluster peer status
----------------------------------
(peers = 3 + localhost maakt 4 ;-)
# gluster volume create testvolume replica 2 172.17.0.2:/bricks/brick01 172.17.0.3:/bricks/brick01 172.17.0.4:/bricks/brick01 172.17.0.5:/bricks/brick01 force
# gluster volume start testvolume ; gluster volume info testvolume
----------------------------------
Volume Name: testvolume
Type: Distributed-Replicate
Volume ID: e5536d11-77ee-40a5-9282-e4223979f443
Status: Started
Snapshot Count: 0
Number of Bricks: 2 x 2 = 4
----------------------------------
# mount -t glusterfs 172.17.0.2:/testvolume /media/
# cd /media
# touch {1..9}
# exit
From dockerhost we see the files nicely spread over the bricks
# for f in 1 2 3 4 ; do echo "gluster_${f}" ; docker exec -it gluster_${f} ls /bricks/brick01 ;done
------------------------------------------------------------------------
gluster_1
1 5 7 8 9
gluster_2
1 5 7 8 9
gluster_3
2 3 4 6
gluster_4
2 3 4 6
---------------------------------------------------------------------------------
Logon op gluster_3
# docker exec -it gluster_3 /bin/bash
# rm -rf /bricks
- wacht ff -
# gluster volume status
----------------------------------------------------------------------------
Status of volume: testvolume
Gluster process TCP Port RDMA Port Online Pid
------------------------------------------------------------------------------
Brick 172.17.0.2:/bricks/brick01 49152 0 Y 210
Brick 172.17.0.3:/bricks/brick01 49152 0 Y 151
Brick 172.17.0.4:/bricks/brick01 N/A N/A N N/A <----- gone
Brick 172.17.0.5:/bricks/brick01 49152 0 Y 152
----------------------------------------------------------------------------
# exit
From dockerhost:
# for f in 1 2 3 4 ; do echo "gluster_${f}" ; docker exec -it gluster_${f} ls /bricks/brick01 ;done
------------------------------------------------------------------------------------
gluster_1
1 5 7 8 9
gluster_2
1 5 7 8 9
gluster_3
ls: cannot access /bricks/brick01: No such file or directory
gluster_4
2 3 4 6
--------------------------------------------------------------------------------------
Logon on gluster_1
# docker exec -it gluster_1 /bin/bash
# gluster volume reset-brick testvolume 172.17.0.4:/bricks/brick01 start
#This is the moment to swap the md3260, but we are using here the next commands:
Create new storage on gluster_3
# docker exec -it gluster_3 mkdir -p /bricks/brick01
# docker exec -it gluster_3 ls /bricks/brick01
Logon on gluster_1
# docker exec -it gluster_1 /bin/bash
# gluster volume reset-brick testvolume 172.17.0.4:/bricks/brick01 172.17.0.4:/bricks/brick01 commit force
[root@svr1035 ~]#
From dockerhost we see the files nicely spread over the bricks
# for f in 1 2 3 4 ; do echo "gluster_${f}" ; docker exec -it gluster_${f} ls /bricks/brick01 ;done
------------------------------------------------------------------------
gluster_1
1 5 7 8 9
gluster_2
1 5 7 8 9
gluster_3
2 3 4 6
gluster_4
2 3 4 6
---------------------------------------------------------------------------------
Welllll .. kinda .. blender using cloth simulation.
I started using cloth simulation to get a blanket “natural/using folds, so not perfect” on a bed scene.
Very cool
A little diagram explaining what i’m using to get a secure mqtt owntracks setup. (reinstall)
Mobile connects with certificates and password to my server in DMZ using port 8883 (no sockets activated at the moment)
Mqtt server in DMZ connects via a bridge to my internal mqtt server. (Both Mosquitto)
Node-red using a worldmap plugin displays the current position of my mobile. Coordinates are stored in a InfluxDB and geolocation is being used to activate/de-activate other node-red nodes.
Start with downloading :
https://github.com/owntracks/tools/blob/master/TLS/generate-CA.sh
Get some environment stuff into place
export IPLIST="83.161.x.x 192.168.1.1 10.12.1.1"
export HOSTLIST="internalhostname.my.lab henriaanstoot.nl mqtt.henriaanstoot.nl"
Generate Certificates and client certificates.
./generate-CA.sh # creates ca.crt and server.{key,crt}
./generate-CA.sh mqttserver # creates server certs
./generate-CA.sh client workstation # creates client certs
./generate-CA.sh client mobile # creates mobile cert
NOTE:
My workstation client is MQTT-explorer, no need to change anything
For owntracks on your mobile you need a P12 certicate, thats a combined cert+key certificate.
openssl pkcs12 -export -in mobile.crt -inkey mobile.key -out mobile.p12
Check certs with
openssl x509 -noout -text -in server.crt | grep DNS:
Download p12 and ca.crt to you mobile.
MQTT-explorer, add a new connection and select workstation.crt ca.crt and workstation.key
Create a mqtt password file
mosquitto_passwd -c /etc/mosquitto/passwordfile firstuser
mosquitto_passwd -b /etc/mosquitto/passwordfile nextuser
TLS mosquitto example, change where needed. (this is a multi setup, portforward ONLY 8883 to this instance)
1883 is only for internal bridge communication.
# Place your local configuration in /etc/mosquitto/conf.d/
#
# A full description of the configuration file is at
# /usr/share/doc/mosquitto/examples/mosquitto.conf.example
pid_file /var/run/mosquitto.pid
persistence true
persistence_location /var/lib/mosquitto/
# Plain MQTT protocol
listener 1883
# End of plain MQTT configuration
# MQTT over TLS/SSL
listener 8883
cafile /etc/mosquitto/certs/ca.crt
certfile /etc/mosquitto/certs/server.crt
keyfile /etc/mosquitto/certs/server.key
allow_anonymous false
password_file /etc/mosquitto/passwords
# End of MQTT over TLS/SLL configuration
listener 9001
protocol websockets
# End of plain Websockets configuration
# WebSockets over TLS/SSL
listener 9883
protocol websockets
cafile /etc/mosquitto/certs/ca.crt
certfile /etc/mosquitto/certs/server.crt
keyfile /etc/mosquitto/certs/server.key
log_dest file /var/log/mosquitto/mosquitto.log
#include_dir /etc/mosquitto/conf.d
connection bridge-01
address INTERNALIP:1883
topic owntracks/# both 0
Plain internal ‘open’ mosquitto setup only needs bridge config
connection bridge-01
address TLSMOSQUITTOINSTANCE-IP:1883
topic # in 2
Made a generic sms sender, for check_mk monitoring + sending password of accounts.
You can send sms by filling in a form, or using a url like:
http://smsgateway.local:8080/sms/0612341234/Message%20met%20spaties
It uses a Raspberry and a sim800L module.
Remove pin from simcard fix:
sudo minicom -b 115000 -o -D /dev/serial0
AT+CPIN?
+CPIN: SIM PIN
AT+CPIN=0000
OK
AT+CLCK=”SC”,0,”0000″
OK
AT+CPIN?
+CPIN: READY
Cron
@reboot sh /home/pi/launcher.sh
Launcher
cat /home/pi/launcher.sh
#!/bin/bash
cd /home/pi
while true; do
/usr/bin/python newapi.py
done
newapi.py (uses flask)
import serial
import RPi.GPIO as GPIO
import os, time
import sys
from flask import Flask, render_template, request
app = Flask(__name__)
from flask_wtf import FlaskForm
from wtforms import StringField, PasswordField, BooleanField, SubmitField, TextAreaField
from wtforms.validators import DataRequired
from flask import render_template
app.config['SECRET_KEY'] = 'you-will-never-guess'
class FormForm(FlaskForm):
telnumber = StringField('telnumber', validators=[DataRequired()])
messagepart = TextAreaField('Text', render_kw={"rows": 5, "cols": 20})
submit = SubmitField('Send Sms')
@app.route("/sms/<number>/<message>")
def action(number, message):
num = number.encode()
mes = message.encode()
GPIO.setmode(GPIO.BOARD)
# Enable Serial Communication
port = serial.Serial("/dev/serial0", baudrate=9600, timeout=1)
# Transmitting AT Commands to the Modem
# '\r\n' indicates the Enter key
port.write('AT'+'\r\n')
rcv = port.read(10)
print rcv
time.sleep(1)
#port.write('ATE0'+'\r\n') # Disable the Echo
#rcv = port.read(10)
#print rcv
#time.sleep(1)
port.write('AT+CMGF=1'+'\r\n') # Select Message format as Text mode
rcv = port.read(10)
print rcv
time.sleep(1)
port.write('AT+CNMI=2,1,0,0,0'+'\r\n') # New SMS Message Indications
rcv = port.read(10)
print rcv
time.sleep(1)
port.write('AT+CSCS="GSM"'+'\r\n')
rcv = port.read(10)
print rcv
time.sleep(1)
# Sending a message to a particular Number
port.write('AT+CMGS="'+num+'"\r\n')
rcv = port.read(10)
print rcv
time.sleep(1)
port.write(mes+'\r\n') # Message
rcv = port.read(10)
print rcv
port.write("\x1A") # Enable to send SMS
for i in range(10):
rcv = port.read(10)
print rcv
return 'OK'
@app.route("/form")
def form():
form = FormForm()
return render_template('web.html', title='Web Sms', form=form)
@app.route('/data', methods = ['POST', 'GET'])
def data():
if request.method == 'GET':
return "The URL /data is accessed directly. Try going to '/form' to submit form"
if request.method == 'POST':
telnumber = request.form['telnumber']
messagepart = request.form['messagepart']
num = telnumber.encode()
mes = messagepart.encode()
GPIO.setmode(GPIO.BOARD)
# Enable Serial Communication
port = serial.Serial("/dev/serial0", baudrate=9600, timeout=1)
# Transmitting AT Commands to the Modem
# '\r\n' indicates the Enter key
port.write('AT'+'\r\n')
rcv = port.read(10)
print rcv
time.sleep(1)
#port.write('ATE0'+'\r\n') # Disable the Echo
#rcv = port.read(10)
#print rcv
#time.sleep(1)
port.write('AT+CMGF=1'+'\r\n') # Select Message format as Text mode
rcv = port.read(10)
print rcv
time.sleep(1)
port.write('AT+CNMI=2,1,0,0,0'+'\r\n') # New SMS Message Indications
rcv = port.read(10)
print rcv
time.sleep(1)
port.write('AT+CSCS="GSM"'+'\r\n')
rcv = port.read(10)
print rcv
time.sleep(1)
# Sending a message to a particular Number
port.write('AT+CMGS="'+num+'"\r\n')
rcv = port.read(10)
print rcv
time.sleep(1)
port.write(mes+'\r\n') # Message
rcv = port.read(10)
print rcv
port.write("\x1A") # Enable to send SMS
for i in range(10):
rcv = port.read(10)
print rcv
return '<a href="/form">Nog een SMS sturen</a>'
@app.route("/checkmk")
def checkmk():
# Enable Serial Communication
port = serial.Serial("/dev/serial0", baudrate=9600, timeout=1)
port.write('AT'+'\r\n')
rcv1 = port.read(30)
time.sleep(1)
port.write('AT+CPAS'+'\r\n')
rcv2 = port.read(30)
time.sleep(1)
port.write('AT+CGREG?'+'\r\n')
rcv3 = port.read(30)
time.sleep(1)
port.write('AT+CGATT?'+'\r\n')
rcv4 = port.read(30)
time.sleep(1)
port.write('AT+CSQ'+'\r\n')
rcv5 = port.read(30)
time.sleep(1)
return 'OK of niet' + rcv1 + rcv2 + rcv3 + rcv4 + rcv5
if __name__ == "__main__":
app.run(host='0.0.0.0', port=8080, debug=True)
I did the donut in Blender!
A few days later i used blender tracking to move around a (incomplete render) of the donut.
I’ve learned a lot by following YT blender tutorials
Printable tracker marks ( https://www.herma.nl/kantoor-thuis/gratis-software/etiketten-assistent-online/etiketten-assistent-online/ )
Herma round labels
I used OpenCV to track my face, and draw something that moves with me when i move.
Just a prove of concept. I will post used code when i find it back.