Last Updated or created 2024-02-10
Step 1 : Convert movie to png’s
ffmpeg -i mymovie.mp4 %04d.png
Step 2 : Run script in same directory
#!/bin/bash #set -x f=MAE numba=$(ls *png | wc -l) numbastart=$(( $numba - 10)) numbapadding=$( printf "%04d\n" $numba) numbapaddingstart=$( printf "%04d\n" $numbastart) echo "$f " mkdir -p images/$f mkdir -p metric/$f for x in $(seq -w 1 $numbapaddingstart) ; do a=$(( $x + 10)) for y in $(seq -w $a $numbapadding) ; do compare -fuzz 20% -verbose -metric $f $x.png $y.png images/$f/$x-$y.png 2> metric/$f/$x-$y.txt echo -n "." done done echo ""
Step 3 : There are metric stats in a subdirectory, let’s find the most matching parts (top 10)
orgpwd=$PWD : > /tmp/top10 more metric/MAE/* | grep all | awk '{ print $2 }' | cut -f1 -d. | sort -n |head | while read ; do grep -H all metric/MAE/* | cut -f1,2 -d. | grep " $REPLY" >> /tmp/top10 done cat /tmp/top10 | cut -f3 -d/ | cut -f1 -d. | while read part ; do echo mkdir -p "$part" startpart=$(echo $part | cut -f1 -d-) endpart=$(echo $part | cut -f2 -d-) for file in $(seq -w $startpart $endpart) ; do echo cp 0${file}.png $part/ done echo cd "$part" echo ffmpeg -y -framerate 30 -pattern_type glob -i \'*.png\' -c:v libx264 -pix_fmt yuv420p out.mp4 echo cd $orgpwd done
Run above script as ./script.sh > mybash.sh
This generates a bash file, check the contents and run using
“bash mybash.sh”
Last step : There are 10 movies in subdirs which should contain the best looping parts.
check these with: (use CTRL-Q in vlc to stop looping and go to the next file
ls */out.mp4 | while read movie ; do vlc -L $movie ; done
Example loop, made with above
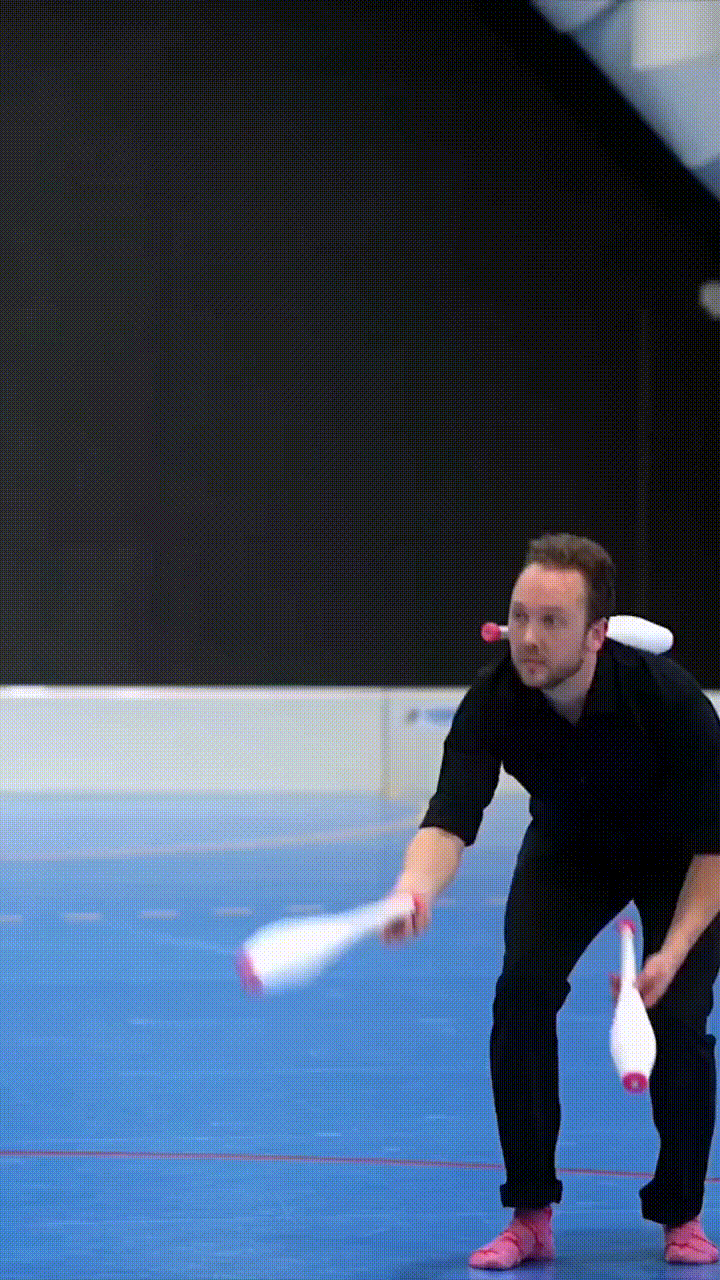