Last Updated or created 2023-08-24
A work in progress – see followup
pip3 install python-vlc && pip3 install PySimpleGUI
Kodi movie NFO file editor test
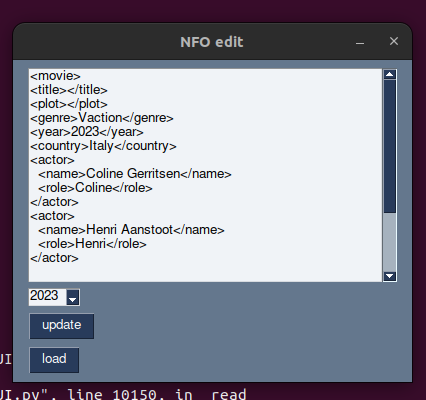
And a player in python
Some example code
from pathlib import Path import PySimpleGUI as sg import vlc from sys import platform as PLATFORM sg.theme('DarkBlue') def btn(name): return sg.Button(name, size=(6, 1), pad=(1, 1)) b1=sg.Button("WRITE NFO") b2=sg.Button("Exit") layout = [[sg.Input(key='-VIDEO_LOCATION-', visible=False, enable_events=True), sg.FileBrowse(file_types=(("MP4 Files", "*.mp4"),))], [sg.Image('', size=(600, 480), key='-VID_OUT-')], [btn('mute'), btn('play'), btn('skip 5sec'), btn('pause'), btn('stop'), btn('snap')], [sg.Text('Load media to start', key='-MESSAGE_AREA-')], [sg.Slider(range=(0, 100), default_value=5, expand_x=True, enable_events=True, orientation='horizontal', key='-SL-')], [[b1, b2]]] window = sg.Window('Mini NFO generator', layout, element_justification='center', finalize=True, resizable=True) window['-VID_OUT-'].expand(True, True) inst = vlc.Instance() list_player = inst.media_list_player_new() media_list = inst.media_list_new([]) list_player.set_media_list(media_list) player = list_player.get_media_player() if PLATFORM.startswith('linux'): player.set_xwindow(window['-VID_OUT-'].Widget.winfo_id()) else: player.set_hwnd(window['-VID_OUT-'].Widget.winfo_id()) player.audio_set_mute(True) while True: event, values = window.read(timeout=1000) if event == sg.WIN_CLOSED: break if event == 'play': list_player.play() if event == 'pause': list_player.pause() if event == 'stop': list_player.stop() if event == 'snap': list_player.pause() newname = values['-VIDEO_LOCATION-'] + ".png" player.video_take_snapshot(0, newname, 400, 300) if event == 'next': player.set_position(0.5) list_player.play() if event == 'mute': player.audio_set_mute(True) if event == '-SL-': player.set_position(int(values['-SL-']/100)) if event == '-VIDEO_LOCATION-': if values['-VIDEO_LOCATION-'] and not 'Video URL' in values['-VIDEO_LOCATION-']: media_list.add_media(values['-VIDEO_LOCATION-']) list_player.set_media_list(media_list) window['-VIDEO_LOCATION-'].update('Video URL or Local Path:') list_player.next() if player.is_playing(): window['-MESSAGE_AREA-'].update("{:02d}:{:02d} / {:02d}:{:02d}".format(*divmod(player.get_time()//1000, 60), *divmod(player.get_length()//1000, 60))) else: window['-MESSAGE_AREA-'].update('Load media to start' if media_list.count() == 0 else 'Ready to play media' ) window.close()