When selling a large part of my computer collection I kept a few odd pieces.
Amongst those was a 8088 DIY machine.
It is a 50PTX3 motherboard with a 8088 compatible CPU
- Power light (Not LED) and connected to power adaptor, not motherboard
- Reset button??? not connected
- Power switch
- Mid center, 5v gnd and 12v
- 5.25″ drive not connected
Tested the power adapter first, a nice 5V and 12V.
Then I plugged the power in the Motherboard add plugged a test ISA card in the slot.
After turning the machine on I saw the Address leds flashing
A NEC D70108C from 1984, which is 8088 pin compatible with Intel 8088 but faster, and has some extra functionality.
The empty socket is for the 8087 Co-processor.
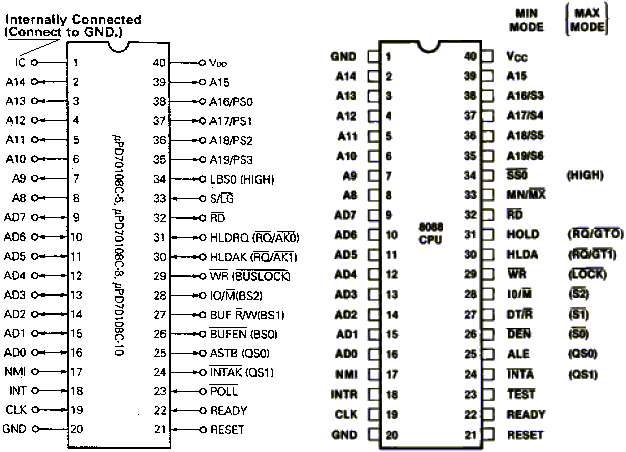
Everything pretty dirty
Adding an 8bit Isa hercules/CGA card.
It starts! .. But there is no Floppy controller (yet)
I’ve dumped the Bios to a file and used a hexeditor to play around.
So that’s why there is a bad checksum.
Installing a ZIF socket (Zero Insertion Force) to make things easier to modify.
Burned GlaBios on the Eprom and now I can continue to play around.
So why? Why this all ..
I want to play around with old 8088 assembly code again, but not as I did before using a Dos machine, but hardcoded into Eproms.
I’ve got 8 banks for ROMs and the source code for GlaBios is available.
In the past Edk and me wrote a boot demo, so it was not utilizing Ms-Dos functions. Maybe i can get some graphical and sound stuff working straight from the Bios.
Some commands:
# Dump the bios to file minipro -w original.rom -p AT2764A@DIP28 xxd and hexdump to view the dump I've used ghex to alter the ROM # Write a new bios to a 28C64 (same Eeprom i've used for the C64 Cartridges) minipro -w /home/henri/Downloads/MYROM -p AT28C64
GlaBios source code:
https://github.com/640-KB/GLaBIOS/blob/main/src/GLABIOS.ASM
I was planning to disassemble the Phoenix Bios, but it’s quite hard to differentiate between code and data, there are no named pointers and you have to interpret every line of code.
So GlaBios it is ..
First code to look at:
This is after the whole post reset. There is a reset pointer at ffff:fffe Which points to the bootstrap routine, which ends in below machine code. I'm going to plug my own code over here. (See the funny remark about Monster as being displayed in one of above pictures) ;----------------------------------------------------------------------------; ; INT 18 - Unbootable IPL ;----------------------------------------------------------------------------; ; Display a disk boot failure message and wait for a key to cold reboot. ; ; This may be re-vectored to ROM BASIC, if present. ; ; Size: 18 bytes ;----------------------------------------------------------------------------; INT_18 PROC ASSUME DS:_BDA_ABS PRINT_SZ BOOT_FAIL ; print boot failure string XOR AX, AX ; AH = 0 (wait for key) MOV DS, AX ; DS = 0000 MOV WARM_FLAG_ABS, AX ; do a cold boot INT 16H ; wait for key press JMP BOOT ; reboot INT_18 ENDP BOOT ENDP ;----------------------------------------------------------------------------; ; ; END OF BIOS POST/BOOTSTRAP ; ;----------------------------------------------------------------------------; ASSUME DS:_BDA STRINGS PROC ;----------------------------------------------------------------------------; ; Banner Strings ; BANNER_STRINGS PROC IF POST_GLADOS EQ 1 BOOT_BEGIN DB CR, LF DB 'Starting GLaDOS...' NL2_Z DB LF ; two NL's, null term'd ENDIF NL_Z DB CR, LF, 0 ; one NL, null term'd BOOT_FAIL DB 'Disk Boot Fail.' DB ' You monster.' NL2_ANY_KEY DB LF NL_ANY_KEY DB CR, LF ANY_KEY DB 'Press the Any Key' DB '...'
Assembly stuff
I’ve got Dosbox installed on my machine.
git clone https://github.com/640-KB/GLaBIOS.git
I copied MASM.EXE and LINK.EXE in the GLaBios src directory.
edit make.bat
change
MASM GLABIOS;
into
MASM /DVER_DATE=”05/24/23″ /DARCH_TYPE=”T” /DCPU_TYPE=”V” GLABIOS;
start dosbox
mount c: /home/henri/projects/
c:
cd glabios/src
make.bat